In this article, we’ll explore how to generate and verify JSON Web Tokens (JWTs) in Rust using the jsonwebtoken
crate. While the library offers support for a range of cryptographic algorithms, we’ll focus specifically on the HS256 and RS256 algorithms.
Before diving into the implementation details, let’s take a moment to understand how these algorithms work and why RS256 is generally considered more secure than HS256.
HS256 (HMAC with SHA-256) is a symmetric algorithm that relies on a shared secret key to generate and verify the JWT signature. This key must be known to both parties involved in generating and verifying the JWT.
On the other hand, RS256 (RSA Signature with SHA-256) is an asymmetric algorithm that uses a public-private key pair. The JWT signature is generated using the private key, and the signature can be verified using the corresponding public key.
While anyone can verify the authenticity of the JWT using the public key, only the party with the private key can generate a valid signature. This makes RS256 more secure than HS256 because it’s harder for an attacker to guess the private key compared to a shared secret.
While we won’t cover every aspect of JWTs in this article, such as their structure and the different algorithms used for generating and verifying signatures, I’ll provide some helpful resources at the end of the article to help you continue your learning.
Our main focus here is on demonstrating how to use the HS256 and RS256 algorithms to sign and verify JWTs in Rust using the jsonwebtoken
crate. By the end of this article, you should have a practical understanding of how to work with JWTs in your own Rust projects!
More practice:
- RESTful API in Rust using Axum Framework and MongoDB
- Rust CRUD API Example with Axum Framework and MySQL
- Rust CRUD API Example with Axum and PostgreSQL
- Create a Simple API in Rust using the Axum Framework
- Build a Frontend Web App in Rust using the Yew.rs Framework
- Frontend App with Rust and Yew.rs: User SignUp and Login
- Rust – How to Generate and Verify (JWTs) JSON Web Tokens
- Rust and Actix Web – JWT Access and Refresh Tokens
- Rust and Yew.rs Frontend: JWT Access and Refresh Tokens
- Rust – JWT Authentication with Actix Web
- Build a Simple API with Rust and Rocket
- Build a CRUD API with Rust and MongoDB
- Implement Google and GitHub OAuth2 in Rust Frontend App
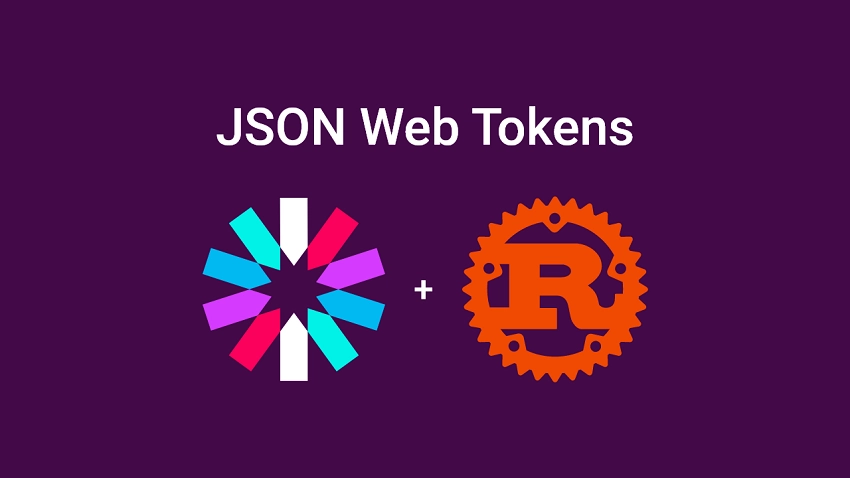
Run a Rust Project Built using HS256 Algorithm
If you are interested in learning more about how the HS256 algorithm can be used to implement authentication in a Rust application, you may refer to the article titled ‘JWT Authentication in Rust using Axum Framework‘. However, if you want to quickly try out a demo of the project without having to write any code, you can follow the steps outlined below:
- Download or clone the Rust Axum JWT authentication project from its GitHub repository at https://github.com/wpcodevo/rust-axum-jwt-auth. Once you have the source code, open it in your preferred IDE or text editor.
- In the root directory’s terminal, start a PostgreSQL server in a Docker container by executing the command
docker-compose up -d
. - Install the SQLX CLI tool by running the command
cargo install sqlx-cli
and then apply the PostgreSQL database’s “up” migration script using the commandsqlx migrate run
. - Install all the required crates and build the project by running the command
cargo build
. - Start the Axum HTTP server by running the command
cargo run
in the terminal. - To test the JWT authentication flow, import the
Rust HS256 JWT.postman_collection.json
file into Postman or the Thunder Client extension in Visual Studio Code. This file contains pre-defined HTTP requests for each of the API endpoints, making it easy to test the application without manually creating the request bodies and URLs.
Getting Started with a Rust Project that Uses RS256 Algorithm
If you’re interested in learning how to implement secure authentication using the RS256 algorithm in Rust, you can check out the detailed guide titled ‘Rust and Axum Framework: JWT Access and Refresh Tokens‘. However, if you’d like to quickly set up the project and see it in action, follow the steps outlined below:
- Download or clone the Rust Axum RS256 JWT project from its GitHub repository (https://github.com/wpcodevo/rust-axum-jwt-rs256) and open the source code in your preferred code editor.
- Launch the PostgreSQL, Redis, and pgAdmin servers in their respective Docker containers by running the command
docker-compose up -d
in the project’s root directory terminal. - Install the SQLx command-line utility by running
cargo install sqlx-cli
if you haven’t already done so. Then, push the migration script to the PostgreSQL database by runningsqlx migrate run
. - Install the required crates and build the project by running the command
cargo build
. - Start the Axum HTTP server by running the command
cargo run
. - To test the Axum RS256 JWT authentication flow, import the Postman collection named
Rust_JWT_RS256.postman_collection.json
into your Postman client or the Thunder Client VS Code extension. This collection includes pre-defined requests, their respective HTTP methods, and the necessary request bodies for the POST and PATCH requests.
How to Sign and Verify JWTs using HS256 Algorithm
In this section, we will cover the essential steps for signing and verifying JWTs using the HS256 algorithm. Fortunately, this process is straightforward, so we won’t go into every detail of the jsonwebtoken
crate’s properties and methods. Instead, we’ll focus on the key steps for signing and verifying your JWTs with HS256.
Sign JWTs using the HS256 Algorithm
To sign a JWT with the HS256 algorithm using the jsonwebtoken
crate in Rust, there are a few steps we need to follow. First, we need to define the token claims. These claims are predefined by the JWT standard, but we can also include our own custom claims. In Rust, we create a struct to hold the fields. Some common fields in a JWT claim include:
"iss" (Issuer)
: this issuer of the token"sub" (Subject)
: the subject of the token"aud" (Audience)
: the audience for the token"exp" (Expiration Time)
: the expiration time of the token"iat" (Issued At)
: the time the token was issued"nbf" (Not Before)
: the time before which the token is not valid
We can name the struct TokenClaims or Claims. The struct must implement the serde::Serialize
trait since the jsonwebtoken::encode()
function expects a type that implements this trait for the token claims.
The second step is to define the JWT secret, which is typically stored in an environment variable. With the JWT secret and claims in place, we can create an instance of the TokenClaims
struct with the required values for the fields.
Finally, we can call the jsonwebtoken::encode()
function, which will encode the header and claims and sign the payload using the HS256 algorithm.
use serde::{Serialize, Deserialize};
use jsonwebtoken::{encode, EncodingKey, Header};
#[derive(Debug, Serialize, Deserialize)]
pub struct TokenClaims {
pub sub: String,
pub iat: usize,
pub exp: usize,
}
let secret = "my_ultra_secure_jwt_secret";
let now = chrono::Utc::now();
let iat = now.timestamp() as usize;
let exp = (now + chrono::Duration::minutes(60)).timestamp() as usize;
let claims: TokenClaims = TokenClaims {
sub: user.id.to_string(),
exp,
iat,
};
let token = encode(
&Header::default(),
&claims,
&EncodingKey::from_secret(secret.as_ref()),
)
.unwrap();
Calling the Header::default()
function will return a JWT header with the default algorithm of HS256. Once we’ve provided the required arguments to the jsonwebtoken::encode()
function, the function will return a Result
containing the token that we can unwrap to get the token.
If your IDE or text editor supports IntelliSense, you can hover over each property and method to get a quick summary of what it does. Alternatively, you can visit the official jsonwebtoken
crate documentation at https://docs.rs/jsonwebtoken/latest/jsonwebtoken/ to learn more about the available features and usage details.
Verify JWTs using the HS256 Algorithm
Now, let’s take a closer look at how we can extract the token claims by decoding and validating the JWT using the HS256 algorithm. Luckily, the jsonwebtoken
crate provides a handy decode()
function that can do just that.
To use this function, we need to make sure that our TokenClaims struct implements the serde::Deserialize
trait since the jsonwebtoken::decode()
function requires a type that implements this trait.
It’s worth noting that the jsonwebtoken::decode()
function will return an error if the signature is invalid or the claims fail validation. Therefore, we need to handle these cases properly to prevent our application from crashing.
To decode and validate the JWT, we can simply call the jsonwebtoken::decode()
function and pass in the token to decode, the secret key to use for decoding, and a set of validation options as arguments.
use serde::{Serialize, Deserialize};
#[derive(Debug, Serialize, Deserialize)]
pub struct TokenClaims {
pub sub: String,
pub iat: usize,
pub exp: usize,
}
#[derive(Debug, Serialize)]
pub struct ErrorResponse {
pub status: &'static str,
pub message: String,
}
let token = "a.jwt.token".to_string();
let secret = "my_ultra_secure_jwt_secret";
let claims = decode::<TokenClaims>(
&token,
&DecodingKey::from_secret(secret.as_ref()),
&Validation::default(),
)
.map_err(|_| {
let json_error = ErrorResponse {
status: "fail",
message: "Invalid token".to_string(),
};
(StatusCode::UNAUTHORIZED, Json(json_error))
})?
.claims;
In the code snippet above, we made use of the map_err
method to handle any errors that may arise during decoding. In the event of a failure, the ErrorResponse
will be returned, and the question mark operator (?) will pass on any errors that occur during decoding.
How to Sign and Verify JWTs using RS256 Algorithm
In this section, we will cover how to sign JWTs using the RS256 algorithm. However, before proceeding, we need to generate the private and public key pairs. There are several ways to generate these keys, including online tools such as the Online RSA Key Generator website, command-line tools like OpenSSL, or specialized software like PuTTYgen.
If you have the flexibility to choose your preferred method, I suggest using the online tool since it is easy to use and doesn’t require any installation on your system.
Generate the Public and Private Key Pairs Online
Let’s start by generating the asymmetric keys, which consist of a private and a public key. It’s important to note that when generating the keys, a key length of 4096 bits should be used instead of 1024 bits or 2048 bits. This is because the jsonwebtoken
crate has been tightening its security, and using a key length of 1024 bits will result in an error.
To generate the asymmetric keys, follow the steps outlined below:
- Visit the Online RSA Key Generator website and select a key size of 4096 bits. Then, click on the “Generate New Keys” button and wait for a moment as the keys are generated.
- Once the keys are generated, you’ll need to copy the private key and convert it to base64 format using a tool like https://www.base64encode.org/. After encoding the key, paste it as the value of the
ACCESS_TOKEN_PRIVATE_KEY
field in a.env
file. - Similarly, copy the public key corresponding to the private key from the Online RSA Key Generator website. Convert the public key to base64 format using the same tool https://www.base64encode.org/ and paste the resulting base64-encoded key as the value of the
ACCESS_TOKEN_PUBLIC_KEY
field. - To create the private and public keys for the refresh token, repeat the steps mentioned above for generating the access token. Then, encode the private key to base64 format and add it to the
.env
file as the value of theREFRESH_TOKEN_PRIVATE_KEY
field. - Add the base64-encoded public key corresponding to the private key as the value of the
REFRESH_TOKEN_PUBLIC_KEY
field.
Note that we convert the private and public keys to base64 format to prevent unnecessary warnings in the terminal when Docker Compose retrieves credentials from the
.env
file.
After following these steps, your .env
file should look similar to the example below.
.env
ACCESS_TOKEN_PRIVATE_KEY=LS0tLS1CRUdJTiBSU0EgUFJJVkFURSBLRVktLS0tLQpNSUlKS0FJQkFBS0NBZ0VBME05dm5IWExKZFgzbk1hWXM4OHVvd1dRS21NSWRNMXVzbGN1MUhZdW01NWs1RE1yCm9pclBXcjcyQW5uVUhVZDczSmo2b3kzSGtYMmZrU3NGSkpVSitZdlQrL3RSRHpGdHlMWXJrbUxFVnJNbmVjVSsKeis0RHJVYitDdmkwUitXWmorMDRLdU1JdTNjSU5ONjh5ZWtQSjB4VVRQSm04bWNtT1ZGN1NJUVBxRXJKR3NtRgp2dTJZOEZGdmo5VkluK2Z3ZmRBeHJhRTEyem05WlhkWnloL2QvU05wZUgxWkVXYmVnSmhPTUJzWWlLcVhMS3V5Clc5bm5uRld2QUNTbGtoYjFLVlY0UW1TV0FVVnNnMEdTMGo5QlFrVkQ1TEZBVWpndDlzSzVDRWtxRGhpS1pNQXIKVFpWVU12eDcwTHRoZmpRNng0ZXljVEVNeG10dXRqam1kYXRQcmJFWGZleHNqNTRIVHlwSzNwSU1ncW1OVTFjNQpHYVFlcW5CSElNa0o2MTk1cUZ4WE5HejE5c2liQlkzTlpleE5HWmc0bkdGTjdrUW9QR2FWdHMyYXdiVU4xL0JZCnBjN0FpSnh5RFg5SkFweUFSUWgxcmxDVkdXb3daQ05WRkJ4OWNMTjBDeGpyYi9td0sxSkRmMHFmSms3QmpyVHcKTnVzL1k5NUp5TE1JSHNvTlpRYk1uL095N2pmMXVjV3dNUkRnYjhqSDdxa2tCQ2F3OW1md2djZVE0cVBtZzFsMgovMjVmQzh1eGlJdWRZWCtQZjBaSVVkQ09zTDllT2xYYWJGcTA4UG5jUmFuRzBFcHRsNnV6eTVuNi9waHdEK0R0Cmh1RE5ycURoNjVTUy9uU1JEVWRHbGtITms0RlByZGNRK0kraWtBZDM1RnJVb0l3ajRjT0VLa0JyT1Q4Q0F3RUEKQVFLQ0FnQnpCUkN4MnFEZ1lwQldwMzZON1YzL0pwMVcrOTQ0bU1DVk5EanpoM1g4K3E4UWxLOUFVTnlQWEFrZgpMQVNQYkVUcUtzcEZBSDZod2RVWG5kN2pXOFYyMUhNY3BqN3NZNG5adVo4ZXI1RC9RUWhKcDBFR1FGRitMVkRhCnNreDhIaGtNa3RzUnBLVzJ2Y2FqZU4zOVNvZXlXZlZGdlhDL3JkbjhVTW5jRkFLYjdUWUJyMmdnMTdnYkNJQ3YKZGdqZkxGL29yYm52cnBHQUJMb3pIaDh6bTRJb1lrMUN0YWxPVUovWHJnM0RxZWxGdnRJdkpSVEdTNjJ0Qy9XdAoyb0hwaXdQWWxOLzlrbktlbUtOQldlbUtMcFcvNzIrS2xhaWNvWjJRQTRydzZYeGs3MWVzVDc2S3Flc0xldENwCkZjNktPakwybmVUSlBQK1FmTFVyWXdSdlpNSXFKOVBVQjZUR1BIRVpsSmROQml5VlNya2d2S2R1NjllemJZZmgKQkRJeXh2Mnh4Q0pSTFU1VUJXb2I0YWp6RWlQZkhmSkIvUnNrOGdVNGNrc0Z2U0ZhZHpPU1hlNlZEYjNRR3NZNgozdFFlK2xsem5lOFVFWTg1NGg2L0JiRENWbHVEa2UxNTk5Ny8yam9MUnl0U0EySGxXc1N4MW41SFp5ZDZ1a1NpCkd4bXgvNHN6b2NGZ1FYVnhhMTljdVlIZXFSK2haa3FGaC9EYTh5UVNsOWRHYXh4WkF5RWplMzBWdjdIeEcxQ0MKQjM4RjZSUmh5Qm9LSnpRbnRNVlY2YXc2Q2FZMk43YS9hRFBLWjRONU5YY0dDKzZSRHh3b0M5bFNleXRrbkRCago1UWVIZmJMai9mRzhQWUU1NnRSWnNEZGNNVmg4SllDdk1acG1uUW9Qb0lUYU9PenNRUUtDQVFFQTl0bzZFOXhnCmZTa1NJMHpDYUdLNkQzMnBmdFJXbkR5QWJDVXpOYk5rcEJLOHJuSGlXanFJVDQzbVd6empGc0RvNlZwVXpscFQKYVVHWkNHMXc5THpHaWlaNllBd0F4TXBOZERzMFFOemhJNjMzS0tseHd0NGlUaGQ0aG9oYmZqdndGWHkyQ0paWgovUkkyZ1AwUEdvSENURXFMMTgzQklpYnJJR0g4dzY2K0F5cFc2L3cvdENEQ2NReFA1RE45YlNPSmFlQVI0a1NzCjg3REM1bmdNMVhJeVFpSCtvL21zaEpUS3ZhZUVpeTVmM1BaaExJNWZNQlZwN0tWTUNZY3V2NWZ4Y3pHVHZFM1YKcHcxamJmSzRDdG9xemFmK3hrdUk5ZWNjakp4TU5KRGc0QW5CNEpxWm11Y2dQWGJPdEpRR2VHaHZqZlBqTVZHZworTHhzSUFWZE8vRjFtUUtDQVFFQTJJeFNNK1VZOTFoem5vUURSbzV4WWVGS0dUeDhVZ00rWDdycURzTXp6NUVSCkRWKzh5WlNsY29NVjNlcGVSdjFHYlRodEUvTlZ4c1k2SW5yUkVJNHB2WFJqYkxqZDZPVkJYWENsYVl1YWsyV20KV2QxTVo4dDZRMUtVWXBFS0piZVRMN09SUmtibnIzTHhmWGJ2WTRPV1BaQjZyNktoaXljbTFubUNJU0hiMFh5Mwp1WHY1VVZEYVZWdklnS0RkNGhrRGZSWmEzNEZZUDYvcUFzMzkyWkJnclpvbVk0SkFMN2F0RnpmWVVZMUtlamV3CmpJWCtpQmRkdkd0cXQ0ZzYwQkgzQUxCZjJFb0Q4bkluaHRuUWtSd0d5QnRFN1pRVGdCYzRJbm5mR2tMZTRpWDkKQlZaSFgxb0VHWUp3RkVUNk1zUHFwcU8yWDhPT21YRDFFVFhUTUVjOGx3S0NBUUFmMWQwUG1xaEcrL2orM0hObQpDdlY3OGZUZUNueHhBY3grSmY0SXV1NEx5dTdTZ0pWMGxYL200cUlHdWo5L083bk4vbnhaY0lTNVdtQm1HZGNyCmVQMFI3QXgwUHBnS3lSeGNGUmFVRnVoaU5abGVnUnZPeWQ4YXV5UXNGWUhYTWR1d3FiakFPc080UTVVTDVaY0IKRUNNQ3U4cDFObS9sKzZidk1qUHErS3BBdGtFbmhneWhLbWhwTS9GSnVPcEFIUWtud21JTUVGZE54a29jZHZjUQp2LzJEVWVjSk5yWHRFMU5pU2l4cDFyMCtQZmdpU3VvenhVODMyY21Jb1FxQ1l4SWNqUlJFZ0xWQktoVGNwU1RmCklXdkx3aEsxZUNCZHRrU1VUY1AyTTRrTTI3VkpSaWJ4TjBXTko3bFl5STVkRVByeUQ3WUpNa0hVVWxpUGVLR2gKalc1aEFvSUJBQWdWQktSbk1vMVl3Y2Z5eVdTQ3dIeVV1ZjFESXFpMDhra0VZdVAyS1NMZ0dURFVsK2sySVE2cgpFYy9jaFhSRTA3SVQzdzVWa0tnQWtmN2pjcFlabURrMzlOWUQrRlJPNmllZ29xdlR5QXNrU2hja2lVdCticXZBCmswVXlnSnh6dzR5T09TZlVVYVZjdHVLbDQ3MWxGZUJxV2duZ0dnTmxqSytJalhETElMY3EzbmlQeGZoZytpVWgKYmRSUExMalpraVhEQmRVOXNKdC81MDMvZmkvMmtZVXBNYkdaRk9neSt6YllvTHc2ZDhNai9QVGhzMlJFNnZ5egpUYUpYOVVuNndhdEc2ZXphcGxjUUo2V0N6NlA2MWMzMkpwWnZabUxyZXU3ZWVaTXpWN285RExwOFErR3RMR1gvClZrdUxYNE14aUxwN2RiMFJRV3M4cWdqZ1oyZHY0VFVDZ2dFQkFMRjRiNnhRNjJJaCtMaTdsVk9lSWQ5VFVub08KUU1LUVNRN0xlWjJ4TmhCYWRPUEt0ZmJ5U0dGMGZieXZiVWk2czAyVnJpWC93S1V6T2o1WEFUbUZYQVdzYnU1dwo2M1JVR09ua2Z6cjIwWDZJWTVzOS9kdnJWZXFLNkpLdlQyZ0F0dWMwNXNCZzJPaG5CdHh2c0JDekhYVy9YRWJsCktWamVIMUxQTnZMaFNSc3BvT2FFVUhlaHpNN2c1V3FGSXhSQmRlb2J1SWNxQ1J2WjRFZGl6b05ybzVRZXFub3oKMTlyU0VVcTNBMEdIdE5Pb0xuV2Q3ZkZta2NOMEw5S3R0MTdsK2wxV0c3Y2kxVTVuSXBlOXBxZThlUUU2YmNYaApkNnlkdWd3UUpXbUxKSlpMQUs3eFpZdzd1ODhoa3ppZ2pSR2ltWHZ4VTJCMTU5OW5OT2NrNWQ0YXJTRT0KLS0tLS1FTkQgUlNBIFBSSVZBVEUgS0VZLS0tLS0=
ACCESS_TOKEN_PUBLIC_KEY=LS0tLS1CRUdJTiBQVUJMSUMgS0VZLS0tLS0KTUlJQ0lqQU5CZ2txaGtpRzl3MEJBUUVGQUFPQ0FnOEFNSUlDQ2dLQ0FnRUEwTTl2bkhYTEpkWDNuTWFZczg4dQpvd1dRS21NSWRNMXVzbGN1MUhZdW01NWs1RE1yb2lyUFdyNzJBbm5VSFVkNzNKajZveTNIa1gyZmtTc0ZKSlVKCitZdlQrL3RSRHpGdHlMWXJrbUxFVnJNbmVjVSt6KzREclViK0N2aTBSK1daaiswNEt1TUl1M2NJTk42OHlla1AKSjB4VVRQSm04bWNtT1ZGN1NJUVBxRXJKR3NtRnZ1Mlk4RkZ2ajlWSW4rZndmZEF4cmFFMTJ6bTlaWGRaeWgvZAovU05wZUgxWkVXYmVnSmhPTUJzWWlLcVhMS3V5Vzlubm5GV3ZBQ1Nsa2hiMUtWVjRRbVNXQVVWc2cwR1MwajlCClFrVkQ1TEZBVWpndDlzSzVDRWtxRGhpS1pNQXJUWlZVTXZ4NzBMdGhmalE2eDRleWNURU14bXR1dGpqbWRhdFAKcmJFWGZleHNqNTRIVHlwSzNwSU1ncW1OVTFjNUdhUWVxbkJISU1rSjYxOTVxRnhYTkd6MTlzaWJCWTNOWmV4TgpHWmc0bkdGTjdrUW9QR2FWdHMyYXdiVU4xL0JZcGM3QWlKeHlEWDlKQXB5QVJRaDFybENWR1dvd1pDTlZGQng5CmNMTjBDeGpyYi9td0sxSkRmMHFmSms3QmpyVHdOdXMvWTk1SnlMTUlIc29OWlFiTW4vT3k3amYxdWNXd01SRGcKYjhqSDdxa2tCQ2F3OW1md2djZVE0cVBtZzFsMi8yNWZDOHV4aUl1ZFlYK1BmMFpJVWRDT3NMOWVPbFhhYkZxMAo4UG5jUmFuRzBFcHRsNnV6eTVuNi9waHdEK0R0aHVETnJxRGg2NVNTL25TUkRVZEdsa0hOazRGUHJkY1ErSStpCmtBZDM1RnJVb0l3ajRjT0VLa0JyT1Q4Q0F3RUFBUT09Ci0tLS0tRU5EIFBVQkxJQyBLRVktLS0tLQ==
ACCESS_TOKEN_EXPIRED_IN=15m
ACCESS_TOKEN_MAXAGE=15
REFRESH_TOKEN_PRIVATE_KEY=LS0tLS1CRUdJTiBSU0EgUFJJVkFURSBLRVktLS0tLQpNSUlKS0FJQkFBS0NBZ0VBbGEyend2WGFoL3pmdXdWN3lQY0FHTG0rY2ZXaVExUm00QWtFZW1wUjZCNmxTMThrCjhsN2Vub0puTGNLY3pLRXhsY2lQKy9uTk5oQlZuNTc2QXh3RERHdmpoRFdqTHIrZzdiUUhYVmozZVNnaG5HUVIKNlhjMTdJNGRzZXd4NVZIYlNXbitWbEt4UkZ4a0xVQVUyUWFVOTNrTFh3UlVjeFhJMngrNFFZeUkra0JGbXdndgozRjB3QXRqS1hFTmhrcmxiMjNsUTNST2l5enRhQWJwcmVYWFVWbGhwM0tGTjZHb052VFRjbEk0MitoYjlZcndDCnN2SkEwdGVxZzdqSWlOTE1XdW41QVg3VlgxOWI0VEt2TzAzc29BMWM5aDVzRHZ2anRvTmdUV25PUFNFanFqNEEKaGhnK09ZWHF0YmROWk5Ld1F3bVhCMGlzVDdwdTEyZ1g3YWU5OWNaeVhPM0dGTkpkTmcvOURCZDBuNkdQL0ZzcQpCZ2lmdkJqNTVUR3J4VDRmNGJUanYyN0xsNzc4Y1h0U2R1aThwY0dMb0E5R2RlZmViTGJCenN0TzZTMzd2ZkR2CjZNQ1oxdHlINW5aWVAzaE5rZlJ1M1pYbUlUSDVwM01zcUpwcVowUGw5ZDVlWXFidG1LMnpIVUVUT1A3bjJpSVQKR1B0RHVWeTQ3SE9QYmVISDVteTVhYzIwQUNHeUlNeXFCSUo3SXZSVDNBUm9QV1V5T2lZKzZkNXJCZjk5ZkpCdAo0NkJZOFVxZDVrcUFULzFjYjArN0NJTm5zRSszV29xYzVQOW94UW1XbnYvRjRURnQ4RUx4em13WGtmQTVhN3JoCmtKZUZqL3JjMXdCOW5sc3J4MHBsWmxwMW80cFlzQVdGQ1hrNzZCSmZiZmtsUGduTUxwbDcxc2VDekIwQ0F3RUEKQVFLQ0FnQitTT1lJTWVKRkpnZW1CWVJoRkhVU1ozVFZOWWZJQXVnaFVicGpobHpBMlVwaEEwOXE1cnd4UkpqRgpOUk9TV3RZNUo5VERwZ21MK2RBa01yK0I3QnB1V29ERlJYUCt0MU9SK25qVU80SGd5UWxDcC9PczVSV3NGbVBiCmdBckJEb1ZUdFlnUFVRbWJRZENMbFN1QnlGbmJTbGRidlkxNjVBQnBVS1BuT2lrLzZ3WlBQV01VSzlPY00walkKKzBqUndHNU9DRmMvajVla25OamQ2R2xST3ljQ0N1cVdhY29QczVzUDdnL0ZqdyszaGJvWG1jVTFNY3VibUxhWApHRXFwbGlFdys0Tkp6YmM5Rm5tdzBWQ2pXcVd3akZYSW1mWlYxaFJVSXhnWGVKTzNZOFJ4bUlwY21RdTNBTlA4CnFVRTFOY1hkYWJQeFExR09teDkxd3ErZHBnOVFpYnN0cnMxZ00wcnRVSmtTRGhjQ2Z4S1ZVekYzSFNZejZpbk4Kc1ZETjRFRGJjTXdHY3huMWh4ZkZPS0haL1hCcmNWLzA5TllPRTdzRGN2TSt5SzF0SEJvRXVscmh0SmJ5YUUyNwpNR3VCaGovbTF0OGhUN25TemdFRnhackFLWHByTzVMdzJPSWo5UmxoenMzME9GbjVDeU04a1ZNS1MxODRkcmdYCnJ3OEV5KzFxUzE0MVdUQWp0ZE9mRW85Q3kyT1U3SGM0N2pPZjdrSS9vTmdWaGQ2QXNUc01RNFRYai93Ymh1ajIKM1l0THkyeElVVVNyRlNZRU9mNFdHTlZjeHVGakk0SnR2Rk9JRTN4MXE4blJ3NkZabDBITkd5QU9SL2tNWlcydwpKZjZWNyszL05yOXJIeHFRRVBpRWEydGx1UUpxbjV0WU9NcEx3SzNhaWh4ektsUmJpUUtDQVFFQXllcUQwUFVQCkdyeWk1WTRoWG1OcGtaaDE4dldsZ2F3eEllS2YrTGd4eEswTE9ZNDk2NW9kUDFVNTFFRUdZSGxFcVVnWW9TSDkKOFY4dFROd1JwWDF6ZTE0LzdYM2NCWHZsTEEwWDRBdm4vNXFKYm9QRUhncFIvSTZyc1pxYnVHRUFhT3N5ME9abAp0aVJNRHU4VWtkTUZBL3lYYVgycWYrRG42YktIWFRtcXVrc3dhejNmNFI0RUUvNjBheTFJeU9BeERDNlJJSnFaCnhSRXJJcWttZHFzR3k5bmEvQy9RYVlBcDJNRDRjWDRkbmVhU2h6Z2hSMWgrWmROV3h4cWhqV3JwMWRkNmMrWk4KekxQcjY0Q2Fmb3c1OWdwLzJld0xOaEFSc1dyclF6OFhLeHFmUVRnN0ZLQm8zangwNWp0WUlaS0lKV0xLZGdhUwp1UklHSUQ3eGt0cFpmd0tDQVFFQXZjVTdqNGRaTGlCVi84TmwvWkwwTXRDa1pBR2ZzWGw4dU9WQU1IZW1QM3JwCjRkbDFaNnYvb05NRjZJQXVjeWlmelR4dlJLNjg1c3dJTlVmOEFKRGRwZXBDZTFjdG1INEpHZUdMWmhic1lFc3cKOEFydTRSM1BNT0dxS0Y0OEZsbktVQTZhY050TEZmZDJvNnlvY0Y0MCtaeXh3QTdhdVllR0ljNTNEZ2FoYUd4Mwo5bitVOXVLSjYzL0FLNGV3dlhsWmhYNUhzRTdmN0sxb0pxdkRMSXRGS01CSVhwaTJVOUpUME1XRm1jK2tkMTVKCjZONTZvVEppWXplNjg0VzRGbGJqSFd4OFlTQU1ad0xpZGFZOEQzVUEvV1l3czNZWDNncG5oa3gxWUJSL3hMaUoKYW1GM3c0MUpOa2Q4UHNmVXRsSmg3UHBPWEQ3aGZSdzRoNmFGMnlYUVl3S0NBUUF1MzZCR0svMmJxVnJ2aTNVMwpva0JwcWtrSFkvdE9CUmxLMG45c2orWU4wRllnd0dLamhSMXhER25tV2tvT3IxZy9MQnQ3bkphRktDRXVESkNVCktIRmNuRjZlMVc3MFh2U3VxME4xb1kzMENuNEpCOUhKWDMvMDczSHdRd0lQWllWZzFlandFZXhld2tKZDNTYWIKUzYrSVkyVUsrajlRZkhlYUN2WGRzSHR2ei9DbmxLK2FaUXR4VU5tMVg4ZmJ5aC9Zd2g2eXdQRWRqSVRGQVJ1Swp4TjFKQ1lRS3MxYmdodjR2OFd3N2ZKbUhoSFZUcXJZZkIrNGYyVlgxMXJyV1I1R05NUDZlVlVLT1dONVZ4MzhXCkRadVBBSlQ1bEJCdU5vREUvUnNzZTBMM29MQ0R4WGdCcTlOc2RBQjNTaU9GZDZ6ZmNQV3JQSTluSTBZRXlsZnUKVFg0bEFvSUJBRzdhWnVkNXpldDI4aVdjYzlpRFhtak1uaXJaRS9ydEY2RStNWmZlWE52YUpnTkxMeHpuU1VVZAozK2FuOGZwTk1jUUcySXlMY2tkenlodXR1QlJ3aXpsZk5ZU3RNVEpSOVdrTDZvMHhPTlVyTnlRUmp1Y3JyWnRGClIwdWJlSWdwM1ZlVW9EenFyTnJoR29tVDB6VUlvdk5veUNDRHpOcnh3clcrMEtiOTBvMllSeDlUK2FXYVFheXkKaklRaEdHb21GOWcySXhSbmpzREhydjVmK1h2c3d2S0NHQVJDT3NlT0ptM2U1Q01zTzB1TFphdEZRdWNrOG5vNAoxTmxxTkZYQVhaMFRnVGlQS3crRmpObml5RlRUS1VmY3lQZ2NOT2I4dHVxcGdTc2w3bGp3M3p5b1FQaVhjTHZuCldEbW9LNlp4UzBqT0VyWXArVGhISXZLQ29OQ2FMemNDZ2dFQkFJeFdyL3MvSjdIZmovc3hUU2xWamFBNHVKdEsKMTNXaW9kMnB3bFFxai9HanZra3VSYTVZQ0g5YlVNTmFqYXpTVmhYOUtkcURoSVJSTHBaTUNSMXlSUWtGb0NSSgpGbTJJbGJCaFlybWptdzEzRzNkL0xhb1RVWFlIMkZ0YnFEQlUyYmFsMEJTNlZOQkcxZUh4dVRtSys1Sk4waldUCkUzQmhtYnl5SnZjcFZ6RUJ1eXcwY1ROeGJncTcxRWRXYlpILzVRcitJSzk4VDh3NzM5N084dFY5cEZuV2MrcVcKejhWQVNiUEc1aEVJTStUbUlzRDFhV0lrWHRsemdxbTRSaFBob3diTmVMRkVkZ1YvVnc3cjBpRUlDbXFOYjVpTgoraTlEZUlZd25WY0FKZk5hV0dwaStRekd2MGl5Szk1TDI4TncrOUtSSzJVK0VnTWZnUzQ5c1lOMW44cz0KLS0tLS1FTkQgUlNBIFBSSVZBVEUgS0VZLS0tLS0=
REFRESH_TOKEN_PUBLIC_KEY=LS0tLS1CRUdJTiBQVUJMSUMgS0VZLS0tLS0KTUlJQ0lqQU5CZ2txaGtpRzl3MEJBUUVGQUFPQ0FnOEFNSUlDQ2dLQ0FnRUFsYTJ6d3ZYYWgvemZ1d1Y3eVBjQQpHTG0rY2ZXaVExUm00QWtFZW1wUjZCNmxTMThrOGw3ZW5vSm5MY0tjektFeGxjaVArL25OTmhCVm41NzZBeHdECkRHdmpoRFdqTHIrZzdiUUhYVmozZVNnaG5HUVI2WGMxN0k0ZHNld3g1VkhiU1duK1ZsS3hSRnhrTFVBVTJRYVUKOTNrTFh3UlVjeFhJMngrNFFZeUkra0JGbXdndjNGMHdBdGpLWEVOaGtybGIyM2xRM1JPaXl6dGFBYnByZVhYVQpWbGhwM0tGTjZHb052VFRjbEk0MitoYjlZcndDc3ZKQTB0ZXFnN2pJaU5MTVd1bjVBWDdWWDE5YjRUS3ZPMDNzCm9BMWM5aDVzRHZ2anRvTmdUV25PUFNFanFqNEFoaGcrT1lYcXRiZE5aTkt3UXdtWEIwaXNUN3B1MTJnWDdhZTkKOWNaeVhPM0dGTkpkTmcvOURCZDBuNkdQL0ZzcUJnaWZ2Qmo1NVRHcnhUNGY0YlRqdjI3TGw3NzhjWHRTZHVpOApwY0dMb0E5R2RlZmViTGJCenN0TzZTMzd2ZkR2Nk1DWjF0eUg1blpZUDNoTmtmUnUzWlhtSVRINXAzTXNxSnBxClowUGw5ZDVlWXFidG1LMnpIVUVUT1A3bjJpSVRHUHREdVZ5NDdIT1BiZUhINW15NWFjMjBBQ0d5SU15cUJJSjcKSXZSVDNBUm9QV1V5T2lZKzZkNXJCZjk5ZkpCdDQ2Qlk4VXFkNWtxQVQvMWNiMCs3Q0lObnNFKzNXb3FjNVA5bwp4UW1XbnYvRjRURnQ4RUx4em13WGtmQTVhN3Joa0plRmovcmMxd0I5bmxzcngwcGxabHAxbzRwWXNBV0ZDWGs3CjZCSmZiZmtsUGduTUxwbDcxc2VDekIwQ0F3RUFBUT09Ci0tLS0tRU5EIFBVQkxJQyBLRVktLS0tLQ==
REFRESH_TOKEN_EXPIRED_IN=60m
REFRESH_TOKEN_MAXAGE=60
Generate the Asymmetric Keys with OpenSSL
Although I recommend using the online tool to generate the asymmetric keys, you can also generate them using OpenSSL, which is included by default in many Linux distributions. However, you’ll need to install OpenSSL as a standalone binary if you are a Windows user.
Once you generate the keys, be sure to encode them in base64 format before adding them to the environment variables file. To generate a private key of length 4096 and write it to a file called private_key.pem
, run the following command:
openssl genrsa -out private_key.pem 4096
To view the contents of the private_key.pem
file, you can use the command cat private_key.pem
. To generate the corresponding public key, you can use the following command which outputs the key into a public_key.pem
file:
openssl rsa -in private_key.pem -pubout -out public_key.pem
Create Utility Functions to Sign and Verify the Tokens
Now that we have the asymmetric keys for the access and refresh tokens, we’ll create two essential utility functions: generate_jwt_token
and verify_jwt_token
. These functions will respectively use the generated private and public keys to sign and verify JWTs.
But before we do that, we need to define two Rust structs that will hold the metadata and claims of the JWT. The TokenDetails
struct will contain the metadata of the JWT and will be returned by both utility functions. The TokenClaims
struct will define the JWT claims as specified in the JWT standard.
To get started, let’s create a new Rust file called token.rs
inside the src
directory and add the following code to it:
src/token.rs
use base64::{engine::general_purpose, Engine as _};
use serde::{Deserialize, Serialize};
use uuid::Uuid;
#[derive(Debug, Serialize, Deserialize)]
pub struct TokenDetails {
pub token: Option<String>,
pub token_uuid: uuid::Uuid,
pub user_id: uuid::Uuid,
pub expires_in: Option<i64>,
}
#[derive(Debug, Serialize, Deserialize)]
pub struct TokenClaims {
pub sub: String,
pub token_uuid: String,
pub exp: i64,
pub iat: i64,
pub nbf: i64,
}
Sign the Tokens using the Private Keys
Let’s start with the generate_jwt_token
function. It takes in a user ID, a time-to-live (TTL) value for the token, and a private key as input parameters. In this function, we first decode the base64-encoded private key back to a UTF8 string.
After decoding the base64-encoded private key, we create instances of the TokenDetails
and TokenClaims
structs that respectively hold the metadata and claims of the JWT. These structs are then passed to the jsonwebtoken::encode()
function, which generates the JWT using the RS256 algorithm.
Finally, we add the generated token to the TokenDetails
struct and return it from the function. Here’s the code for the function:
src/token.rs
pub fn generate_jwt_token(
user_id: uuid::Uuid,
ttl: i64,
private_key: String,
) -> Result<TokenDetails, jsonwebtoken::errors::Error> {
let bytes_private_key = general_purpose::STANDARD.decode(private_key).unwrap();
let decoded_private_key = String::from_utf8(bytes_private_key).unwrap();
let now = chrono::Utc::now();
let mut token_details = TokenDetails {
user_id,
token_uuid: Uuid::new_v4(),
expires_in: Some((now + chrono::Duration::minutes(ttl)).timestamp()),
token: None,
};
let claims = TokenClaims {
sub: token_details.user_id.to_string(),
token_uuid: token_details.token_uuid.to_string(),
exp: token_details.expires_in.unwrap(),
iat: now.timestamp(),
nbf: now.timestamp(),
};
let header = jsonwebtoken::Header::new(jsonwebtoken::Algorithm::RS256);
let token = jsonwebtoken::encode(
&header,
&claims,
&jsonwebtoken::EncodingKey::from_rsa_pem(decoded_private_key.as_bytes())?,
)?;
token_details.token = Some(token);
Ok(token_details)
}
Verify the Tokens using the Public Keys
To validate JWTs and extract their payloads, we need to implement the verify_jwt_token
function. This function takes a public key and a token as input parameters. The first step is to decode the base64-encoded public key back to a UTF8 string.
After decoding the public key, we create a new instance of the jsonwebtoken::Validation
struct, where we specify the RS256 algorithm used in signing the JWT.
Then, we call the jsonwebtoken::decode()
function, which decodes the JWT using the provided public key and validation parameters. The result is stored in a decoded
variable, which contains the claims of the JWT.
As the ‘sub‘ and ‘token_uuid‘ fields of the TokenClaims
struct are stored as strings, we need to convert them into UUID types. To do this, we use the Uuid::parse_str()
function and assign the parsed values to the ‘user_id‘ and ‘token_uuid‘ variables, respectively.
Finally, we create a new instance of the TokenDetails struct with the parsed user_id
and token_uuid
values, set the expires_in
and token
fields to ‘None‘, and return it from the function.
Here is the implementation of the verify_jwt_token
function:
src/token.rs
pub fn verify_jwt_token(
public_key: String,
token: &str,
) -> Result<TokenDetails, jsonwebtoken::errors::Error> {
let bytes_public_key = general_purpose::STANDARD.decode(public_key).unwrap();
let decoded_public_key = String::from_utf8(bytes_public_key).unwrap();
let validation = jsonwebtoken::Validation::new(jsonwebtoken::Algorithm::RS256);
let decoded = jsonwebtoken::decode::<TokenClaims>(
token,
&jsonwebtoken::DecodingKey::from_rsa_pem(decoded_public_key.as_bytes())?,
&validation,
)?;
let user_id = Uuid::parse_str(decoded.claims.sub.as_str()).unwrap();
let token_uuid = Uuid::parse_str(decoded.claims.token_uuid.as_str()).unwrap();
Ok(TokenDetails {
token: None,
token_uuid,
user_id,
expires_in: None,
})
}
Use the Utility Functions to Sign and Verify JWTs
At this point, we have done 90% of the work by isolating the code required to sign and verify JWTs into a separate module. With this modular approach, we can now easily use the utility functions to sign access and refresh tokens, and validate them as necessary.
Typically, the code for signing JWTs would be placed in a login route handler function, while the code for verifying JWTs would be placed in a middleware function.
Sign the JWT using the RS256 Algorithm
To sign the access and refresh tokens, we need to read the private keys and their respective max-age values from the .env
file. Instead of repeating the code to retrieve the environment variables, we’ll create a utility function called get_env_var() to handle this for us. In a typical Rust project, the code for retrieving environment variables would be in a separate src/config.rs file, but for simplicity, we will keep it in the same file below.
Once we have the RSA keys, we can create a function called generate_token()
that will use the token::generate_jwt_token()
function to sign the JWTs. This allows us to avoid duplicating code for both the access and refresh tokens.
Finally, we will call the generate_token()
function twice, once for the access token and once for the refresh token, to generate both JWTs.
fn get_env_var(var_name: &str) -> String {
std::env::var(var_name).unwrap_or_else(|_| panic!("{} must be set", var_name))
}
let access_token_private_key = get_env_var("ACCESS_TOKEN_PRIVATE_KEY");
let access_token_max_age = get_env_var("ACCESS_TOKEN_MAXAGE");
let refresh_token_private_key = get_env_var("REFRESH_TOKEN_PRIVATE_KEY");
let refresh_token_max_age = get_env_var("REFRESH_TOKEN_MAXAGE");
fn generate_token(
user_id: uuid::Uuid,
max_age: i64,
private_key: String,
) -> Result<TokenDetails, (StatusCode, Json<serde_json::Value>)> {
token::generate_jwt_token(user_id, max_age, private_key).map_err(|e| {
let error_response = serde_json::json!({
"status": "error",
"message": format!("error generating token: {}", e),
});
(StatusCode::INTERNAL_SERVER_ERROR, Json(error_response))
})
}
let access_token_details = generate_token(
user.id,
access_token_max_age,
access_token_private_key.to_owned(),
)?;
let refresh_token_details = generate_token(
user.id,
refresh_token_max_age,
refresh_token_private_key.to_owned(),
)?;
Verify the JWT using the RS256 Algorithm
Now that we have the code for signing the access and refresh tokens, we can move on to implementing the code that will validate and extract their payloads. Our solution is to use the token::verify_jwt_token()
function, which we created earlier.
To get started, we first need to obtain the public keys from the .env file. Once we have the public keys, we will call the token::verify_jwt_token()
function twice, once for the access token and once for the refresh token.
use crate::token;
fn get_env_var(var_name: &str) -> String {
std::env::var(var_name).unwrap_or_else(|_| panic!("{} must be set", var_name))
}
let access_token_public_key = get_env_var("ACCESS_TOKEN_PUBLIC_KEY");
let refresh_token_public_key = get_env_var("REFRESH_TOKEN_PUBLIC_KEY");
let access_token = "a.jwt.access.token".to_string();
let access_token_details =
match token::verify_jwt_token(access_token_public_key.to_owned(), &access_token) {
Ok(token_details) => token_details,
Err(e) => {
let error_response = ErrorResponse {
status: "fail",
message: format!("{:?}", e),
};
return Err((StatusCode::UNAUTHORIZED, Json(error_response)));
}
};
let refresh_token = "a.jwt.refresh.token".to_string();
let refresh_token_details =
match token::verify_jwt_token(refresh_token_public_key.to_owned(), &refresh_token)
{
Ok(token_details) => token_details,
Err(e) => {
let error_response = serde_json::json!({
"status": "fail",
"message": format_args!("{:?}", e)
});
return Err((StatusCode::UNAUTHORIZED, Json(error_response)));
}
};
While I’ve addressed errors in the match blocks using Axum-specific code, it’s worth noting that you’ll want to handle any potential errors resulting from the token::verify_jwt_token()
function in a way that’s appropriate for your chosen web framework.
Helpful Resources
Here’s a list of useful resources that can help you deepen your understanding of JWTs and the associated risks. If you have any additional resources to suggest, feel free to leave them in the comments below and I’ll add them to the list.
- Why JSON Web Tokens (JWT) are Dangerous for User Sessions
- All About Attacking JWT
- What Are Refresh Tokens and How to Use Them Securely
Conclusion
That concludes our guide to generating and verifying JWTs in Rust using the HS256 and RS256 algorithms, as well as exploring various methods for generating RSA keys. I hope you found this tutorial useful and enjoyable. If you have any questions or feedback, please leave them in the comments below. Thank you for reading!
Hi, cool article! Can you make one about PASETO tokens? They are more secure, because as symmetric algos they use both encryption and signing and for asymmetric algo they use Ed25519 which is more secure, than ECDSA and RSA. I suggest read: https://dev.to/techschoolguru/why-paseto-is-better-than-jwt-for-token-based-authentication-1b0c
I appreciate your suggestion about PASETO tokens and the linked article. It sounds like an interesting topic to explore, and I’ll definitely consider it for a future article.
Thank you for sharing your knowledge and resources.
Here is good paseto library for rust: https://github.com/brycx/pasetors. And paseto specification: https://github.com/paseto-standard/paseto-spec
Thank you for sharing!