The warning To load an ES module, set “type”: “module” in the package.json or use the .mjs extension occurs when using ES6 Module import syntax in a NodeJs application without setting the type property in the package.json to module.
To solve this warning you need to make sure you have a package.json file then add a type property and set it to module.
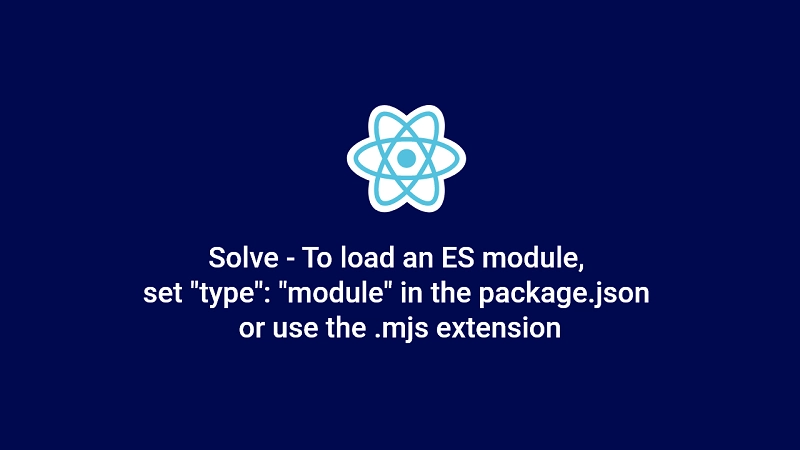
Also, you need to know that all the files you create in a Node application are recognized by the Node runtime as modules.
Create a Package.json file (Optional)
If you don’t have a package.json file yet open your terminal and cd into the working directory and initialize a new Node project with this command.
// For NPM users
npm init -y
// For Yarn users
yarn init -y
Setting the -y flag allows you to say Yes to all the various questions you would have answered when you initialize a project without appending the -y flag.
Also if you haven’t installed the packages you’re trying to import then make sure you install them.
Set type property in Package.json to module
Now you can open the package.json file then add a new property called type and set the value to module.
{
"type": "module"
}
After setting the type property to module in your package.json, you can now use ES6 import and export without experiencing any errors.
import express from 'express';
const app = express();
const port = 5000;
app.listen(port, console.log(`Server started on port: ${port}`));
Another scenario is to set the filename extension to .mjs to implicitly tell Node the file is a module. Don’t need to explicitly set the type property to module in the package.json file if you named your files with the .mjs file extension.
You can use default imports/exports with ES6 modules in your Node projects in the following ways:
Instead of listening to the port in the app.js file I exported the app instance so that I can import it into a server.js file.
import express from 'express';
const app = express();
// ? default export
export default app;
In the above snippets, you can see I used export default to export the app.
Now inside the server.js file, I need to use a named import to get the app instance I exported from the app.js.
// ? default import
import app from './main.js';
app.listen(port, console.log(`Server started on port: ${port}`));
You can see am starting the server in the server.js file instead of the app.js file.
Note: You can have only one default export per file.
When you want to export multiple things from a file then you need to use a named export instead of default export.
Note: You can have named export and default export in the same file.
// ? named export
export function sendMessage(message) {
return `${message} ????`;
}
// ? named export
export function showMessage(message) {
console.log(message);
}
// ? default export
export default function add(a, b) {
return a + b;
}
Inside the server.js file, we can import those functions we exported.
// ? default import
import app from './main.js';
// ? named import
import {sendMessage, showMessage} from './calc'
// ? default import
import add from './calc'
sendMessage('Say Hello')
showMessage('Say Hi')
add(8,9)
app.listen(port, console.log(`Server started on port: ${port}`));
In this article, you learned how to solve the warning To load an ES module, set “type”: “module” in the package.json or use the .mjs extension.