As a developer, mastering your IDE can go a long way to improve your daily productivity.
Visual Studio Code is an open-source programming editor designed and maintained by Microsoft and it comes with great features out of the box.
To become a VSCode power user, it’s recommended to master all the VSCode Tips and Tricks to help you become more productive in all your Python projects.
I don’t think VSCode is particularly the best text editor for Python development since there is another option which is Pycharm.
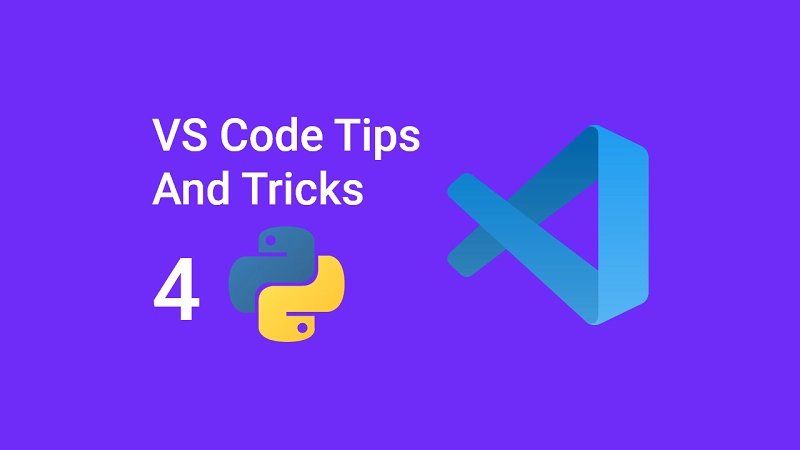
Pycharm is an IDE built for Python development and it has better refactoring and tools that are more geared toward Python project development.
Nevertheless, VS Code can also be powered with extensions to make it perform at the same level as Pycharm.
Pycharm only supports Python whereas VSCode supports a lot of languages and it really works very well for web development. VSCode has great support for TypeScript, NodeJS, React JS, Vue Js, and so on.
It is always recommended to learn and master one IDE and stay with that particular IDE throughout your coding journey.
The moment you make a switch to another IDE, there is a high chance of screwing up the keyboard shortcuts and that is going to be annoying.
So sticking with VS Code even though it’s not one-hundred percent perfect for Python development will be the best choice for you.
In this article, we are going to elaborate on the settings you must use in VS Code and the extensions you need to install to improve your productivity as a Python developer.
In the previous article in this series, 25 VS Code Productivity Tips and Speed Hacks, we talked about 25 VS Code productivity tips and tricks every developer should adopt to improve their productivity.
You can also read:
- Top 21 VS Code Shortcuts Every Programmer Should Master
- Top 10 Best VS Code Extensions for React Developers
Why Use VS Code For Your Python Projects
VSCode might not be one hundred percent ideal for some Python developers but I recommend it to most developers because of two reasons.
- Microsoft’s VS Code is lightweight compared to the robust Pycharm IDE and I can boldly say that it’s much faster than Pycharm.
Pycharm is more robust and becomes more relevant when building complex projects. - VS Code is an open-source IDE and it’s completely free. Pycharm on the otherhand comes in two forms, a community version which is also free and a professional version which is paid.
Terminal Setup
Learning how to work and run scripts in the terminal can be one of the best VSCode tips and tricks you can add to your development toolbox.
VSCode has a built-in terminal and that is what you’ll get when you install it for the first time.
On a Mac computer, the default VSCode terminal is Z shell and on Windows the default VSCode terminal is PowerShell.
There are great extensions that will allow you to customize the default terminal that comes with VSCode to suit your need.
Oh My Zsh
Oh My Zsh is an open-source framework for managing Zsh configuration. It comes with a bunch of helpful functions, helpers, plugins, themes and many more.
The most useful feature of this extension is it shows what Git branch you’re on in the terminal.
When you change the directory “cd” to any of your project directories, it will automatically display that it’s a Git repository and also shows the branch.
iTerm2
iTerm2 is specifically a terminal emulator for macOS that you can use instead of the built-in Mac terminal.
It also has a lot of extra features like having split panels or lots of hotkeys that allow you to bring the terminal onto the screen from wherever you are in any application.
How to change the terminal style in VSCode
VSCode gives you the freedom to completely customize the styles of the terminal which overrides the theme.
After customizing the styles of the terminal in VSCode the terminal will look the same no matter what theme you install.
- Navigate to settings in Visual Studio Code (VS Code)
- On Windows || Mac || Linux: Go to File –> Preferences –> Settings
- Search for “workbench: color customizations” and click on the settings.json file
- Edit or Paste your configuration under the workbench.colorCustomizations if that property already exists else create one.
Visit this website to choose the particular terminal styles you want, this website consist of a lot of color themes you can preview before copying the configuration file.
"workbench.colorCustomizations": {
"terminal.background": "#090300",
"terminal.foreground": "#A5A2A2",
"terminalCursor.background": "#A5A2A2",
"terminalCursor.foreground": "#A5A2A2",
"terminal.ansiBlack": "#090300",
"terminal.ansiBlue": "#01A0E4",
"terminal.ansiBrightBlack": "#5C5855",
"terminal.ansiBrightBlue": "#01A0E4",
"terminal.ansiBrightCyan": "#B5E4F4",
"terminal.ansiBrightGreen": "#01A252",
"terminal.ansiBrightMagenta": "#A16A94",
"terminal.ansiBrightRed": "#DB2D20",
"terminal.ansiBrightWhite": "#F7F7F7",
"terminal.ansiBrightYellow": "#FDED02",
"terminal.ansiCyan": "#B5E4F4",
"terminal.ansiGreen": "#01A252",
"terminal.ansiMagenta": "#A16A94",
"terminal.ansiRed": "#DB2D20",
"terminal.ansiWhite": "#A5A2A2",
"terminal.ansiYellow": "#FDED02"
}
Main Python Extensions You Must Start Using
Python
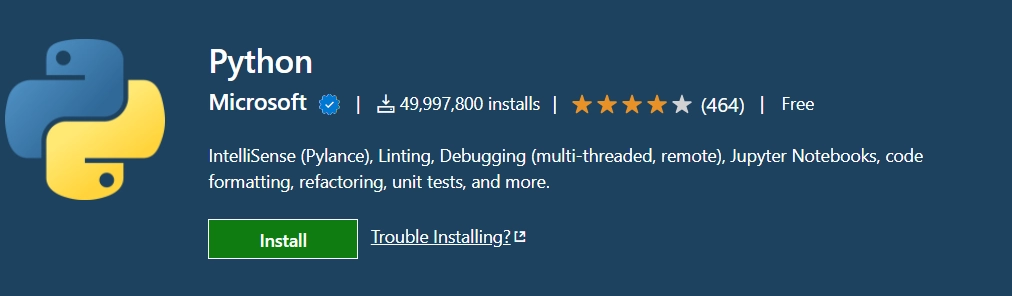
VSCode does not have full support for Python so when you create a .py file it will immediately suggest you install this extension when you open the .py file.
During the installation, this extension will also install additional extensions like Pylance, Jupyter, and Pylint to further take VSCode to a whole new level.
It also supports Jupyter Notebooks and therefore can be classified as the most essential Python extension for VSCode.
This extension is developed and maintained by Microsoft, the same company behind Visual Studio Code itself.
Here are some of the features and benefits you get after installing the Python extension in VSCode:
- Code Linting – Supports add-ons like Pylint or Flake8 for linting your code.
- Code formatting, Auto-activation, Syntax Checking and Auto-completion
- Supports Jupyter Notebooks, Unit test, Pytest and nose test framework.
- Automatically activate and switch between venv, virtualenv, pipenv, pyenv, and conda environments. There will be no need to manually activate the virtual environement yourself.
Demo of how the Python extension works:
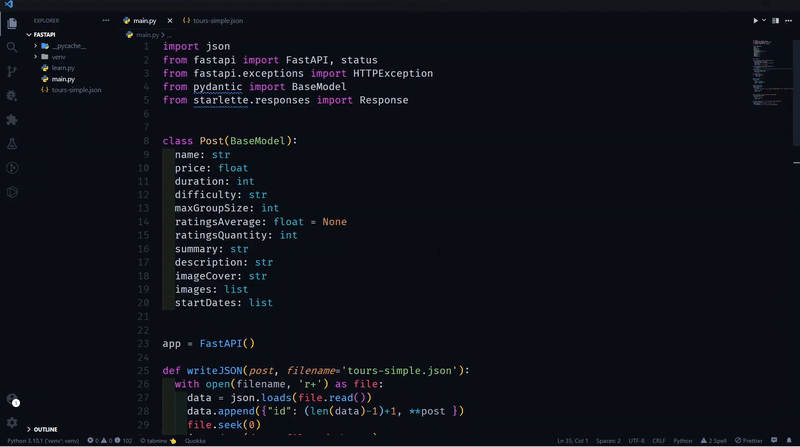
Python Snippets
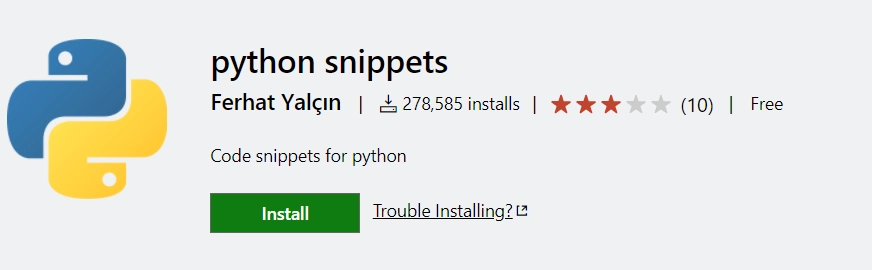
Python Snippets extension can be useful for beginner Python developers who are getting their hands thirty with Python code.
This extension was developed by Ferhat Yalçın and contains built-in Python snippets for strings, class, tuple, dictionaries, sets, and many more.
This extension is beginner-friendly because it provides at least one example for each snippet, saving you a lot of time when writing Python codes.
Python Preview
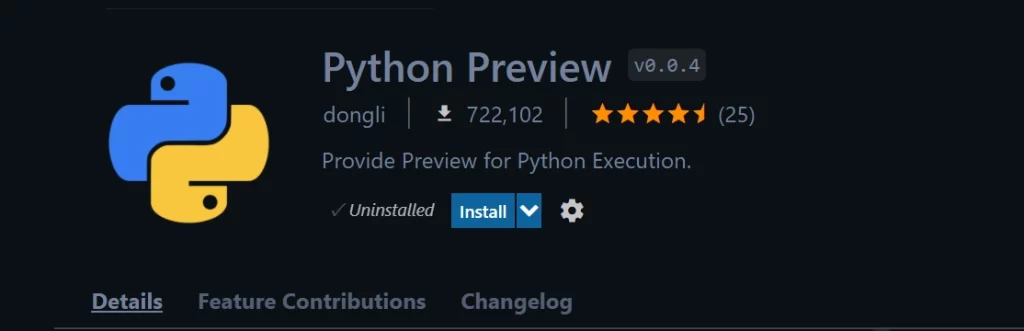
This extension allows you to preview your Python code directly in VS Code editor. If you are looking to find and fix bugs in a visual way then the Python Preview extension is a must-use for you.
This extension transforms your code into graphics and animations to help you visualize and understand the status of your Python code.
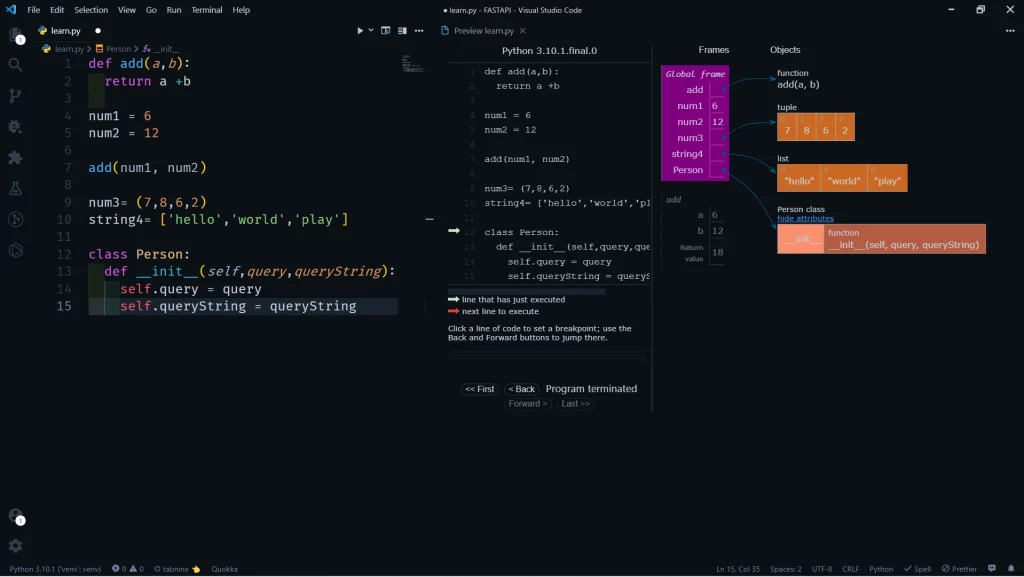
Python Docstring Generator
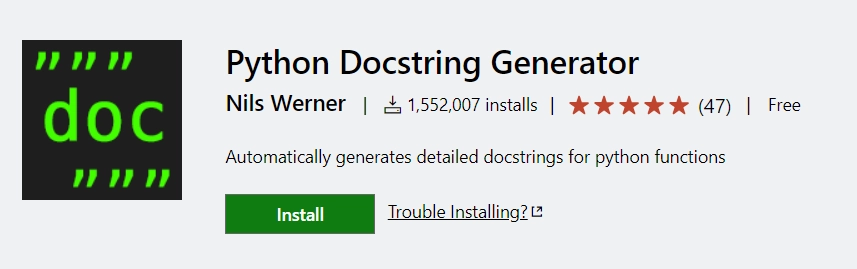
This plugin makes it easy to document your Python code in VS Code by following all the standard formats and has support for parameter types, decorators, Kwargs, args, and errors.
It also auto-creates docstrings, allows for tabbed navigation and when the docstring is generated it can be formatted per the developers’ requirement.
Type Checking
When you write Python programs then basically IntelliSense is going to help you by auto-suggesting the available functions and classes in a particular module when you are importing it into your project.
The Pylance and the linting tools also help you identify issues in your code. For example, you will see an error or warning when trying to import a function or class that doesn’t exist in a module.
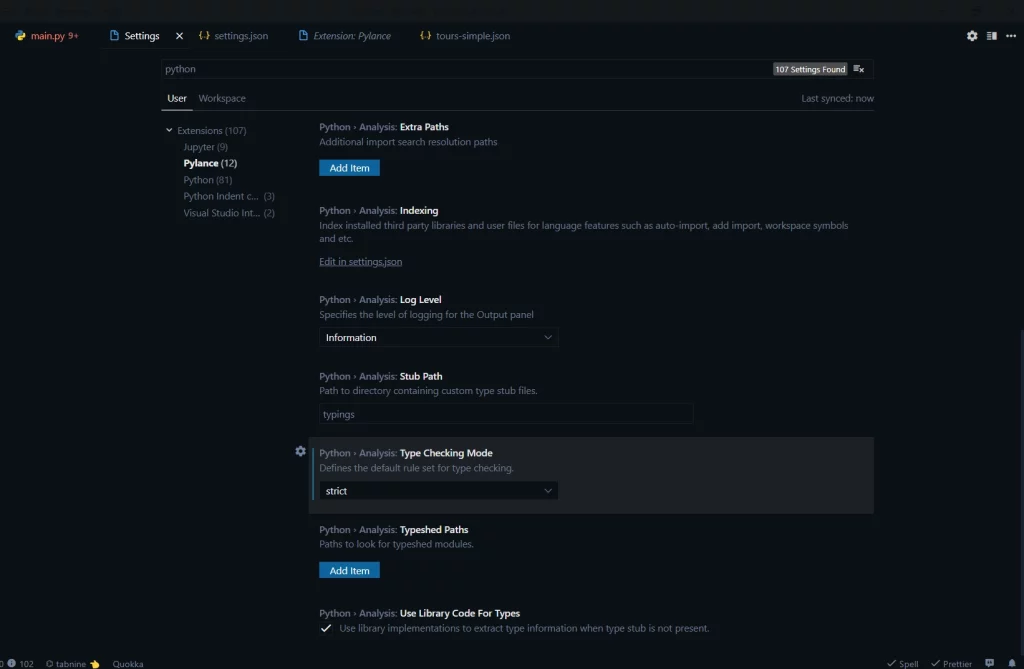
In your VS Code settings, Search for Python and under the Extensions’ dropdown select Pylance and change the Type Checking Mode to strict and this will give errors for any issue that happens with typing.
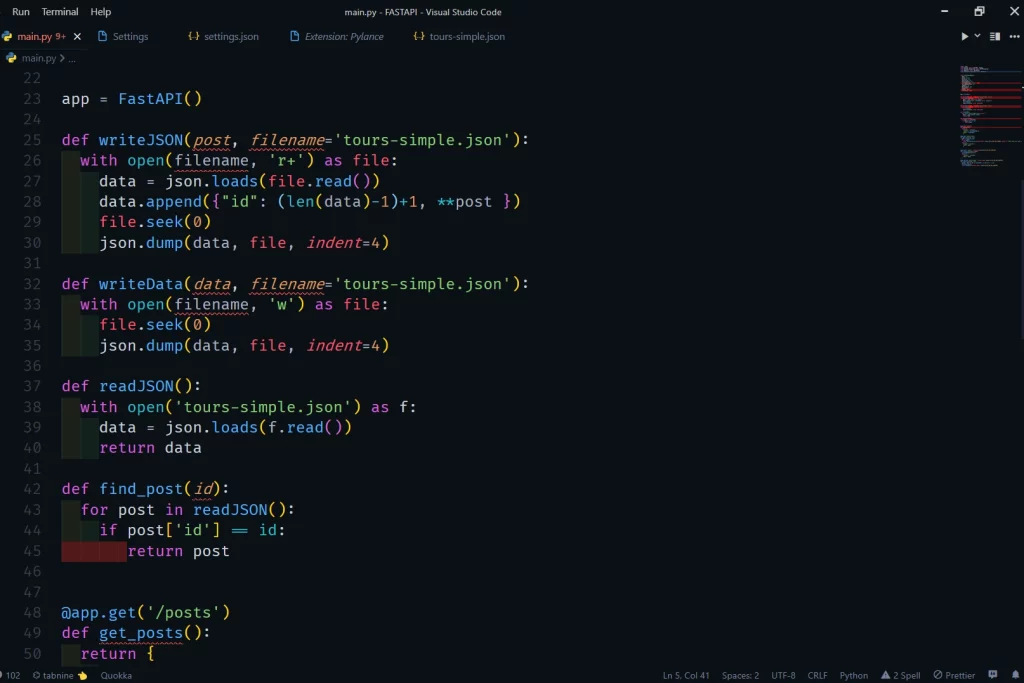
With Type Checking in Pylance, there are three levels, we have “off” mode which actually turns off type checking, we also have “basic” mode which does basic type checking and finally the “strict” mode which performs strict type checking.
The difference between the “basic” and “strict” mode is they have a bunch of different rules which are turned off or on based on whether you in a basic level or strict level.
Depending on the type of project you are working on you can choose different type checking modes.
Code Formatting
There are a couple of settings I have that auto-format my code when I save a file or paste some snippets of code.
There are a good number of formatting providers you can choose from like black, auto pep8 and more.
I normally like black since it’s really simple, so what it does is when you write a piece of code and save the file it will automatically format the code for you so that you don’t have to worry about style anymore.
Vim and Smart Relative Lines
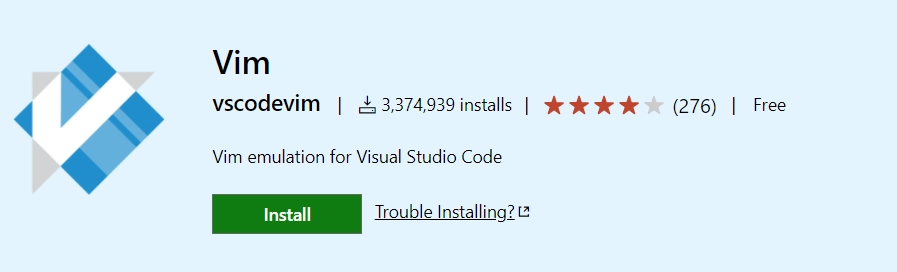
Vim is a better option than Syntastic because it utilizes the async feature in Vim8 and this will help you during syntax checking.
The Vim VS Code emulator plugin is useful for stuff like better syntax highlighting, syntax checking, code completion and proper code refactoring.
There are a lot of possibilities when it comes to Vim and it’s a bit intuitive but once you start playing with it you will get more comfortable with it.
After installing the Vim emulator plugin in VS Code, you can toggle the “vim.smartRelativeLine” to true so that as you navigate up and down it shows the line number and the relative lines numbers up and down the current line that you are at.
VSCode Theme Customization
Visual Studio Code gives you the freedom to customize the editor to suit your style. With more than 1,000 unique stylish themes and color pallets, it can be daunting to find the best one.
I will provide my top 5 VS Code themes you can choose from to make your text editor look more professional.
AI Tools (GitHub Copilot, Tabnine)
AI autocomplete engines are now becoming more popular and a lot of people fear that AI autocompletes engines will replace software developers.
Well, the good news is that these autocomplete engines won’t replace professional software developers since they only make your life easier by performing repetitive tasks which can save you a lot of time.
Keep in mind that these autocomplete engines are here to help, one thing they do is to look up documentation and real-world examples automatically in the background without having to open the browser and search for them.
Here are the most noticeable AI autocomplete engines you can try out to see how beneficial they are when you are coding a program.
GitHub Copilot
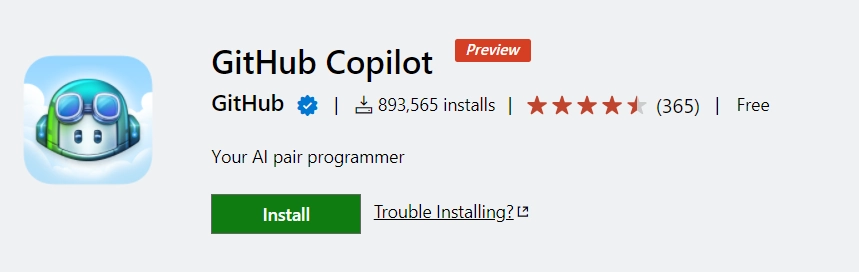
GitHub Copilot is a new tool in the AI autocomplete engine world with the latest AI tools backing it.
According to GitHub, GitHub Copilot is a collaboration between Microsoft and Open AI, the “OpenAI Codex” was trained on publicly available GitHub repositories and natural language, so it understands both human languages and programming.
One downside is it’s only supported in Visual Studio Code.
Tabnine
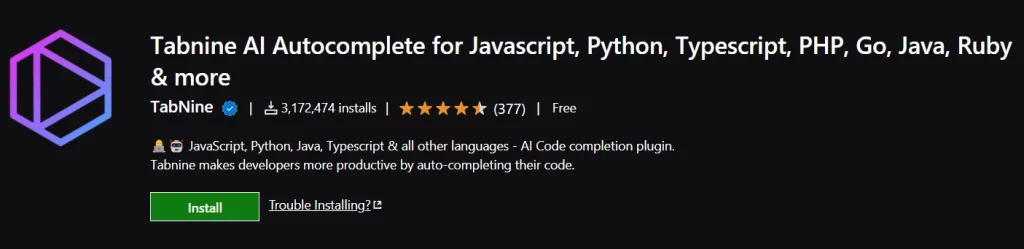
Tabnine has been around since 2019 and it’s actually the AI Code Autocomplete plugin I use in VS Code.
Sometimes I shout “Woow” how does it know what I wanted to type? This saves me a lot of code input and time.
Conclusion
Here are all the best VSCode Tips and Tricks I have been using in my Python projects and I can boldly say that I have improved my productivity after learning those tips and tricks.
Please don’t forget to check the previous tutorial in this series, 25 VS Code Productivity Tips and Speed Hacks which also explains 25 productivity tips and speed hacks you can adopt as a developer to help you become more productive.
Thanks! This was so helpful
I’m glad that the tutorial was useful to you!