Python is undoubtedly one of the most popular programming languages that are being used by a vast majority of companies and private individuals as their primary programming language.
As a beginner, you might have questions like what are Python Modules and how are they useful in Python programming at large. Well, your questions are about to be answered in this article.
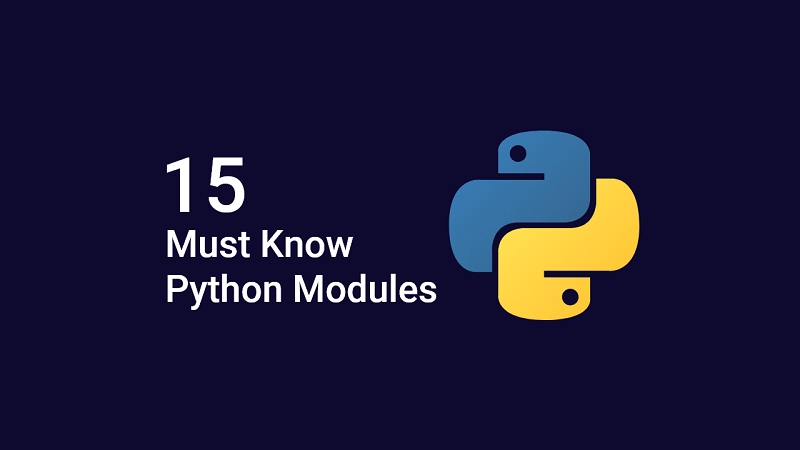
The Python language has shown incredible growth and popularity year by year due to a couple of reasons.
The first is Python is incredibly easy to use and learn because it has simplified syntax that makes it to be easily understood by amateur developers.
Due to its low learning curve, Python codes can be written and executed much faster compared to other programming languages.
The second reason is its efficiency, versatility, and readability.
Python gives developers the freedom to create applications that run in different environments such as desktop applications, mobile applications, web development, hardware programming, and so on.
The third and final reason is Python has a large number of corporate sponsorship, a big community of developers, and more than 200,000 Python packages or libraries.
In one of the tutorials in this series, Essential Python String Methods, we talked about all the essential Python string methods you need to know as a Python developer.
In this article, we will discuss the 15 Python modules you need to know as a Python developer.
I have divided the modules into four different categories to make things easier for us. The categories are Web Development, Data Science, Machine Learning AI, and Graphical User Interface.
Top 15 Most Essential Python Modules for Developers
Web Development Modules
The modules we will start with are those that deal with web development and HTTP/HTTPS requests.
Python is well known for backend web development and therefore a lot of developers all over the world have created different modules to make the building of enterprise-level websites in Python easier.
Requests Module
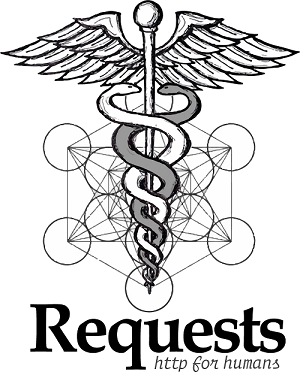
- Summary of what it does: Make HTTP requests
- GitHub Stars: 46,821
- Creator: Kenneth Reitz
- Library Link: https://pypi.org/project/requests/
- GitHub Link: https://github.com/request/request
The Requests module is used to send HTTP requests with ease, it’s very simple, easy to use and is even the most downloaded python package at the time of writing this article.
Create a new folder on your desktop and give it any name of your choice. Also, you need to create a virtual environment to avoid installing the python modules on your actual computer disk.
After opening the folder in your preferred text editor, run this command to install the Requests module in the virtual environment.
pip install requests
The most common way to play with HTTP requests in most programming languages is to use JSONPlaceholder RESTful API.
Run the snippets below to make a GET request to the JSONPlaceholder RESTful API to get all users from the /users endpoint and print the result in the terminal.
import requests
res = requests.get('https://jsonplaceholder.typicode.com/users')
print(res.json())
Some of the popular features of the Requests module include – Chunked HTTP requests, connection pooling, basic authentication, connection timeouts, and support for the SOCKS proxy.
Django Framework
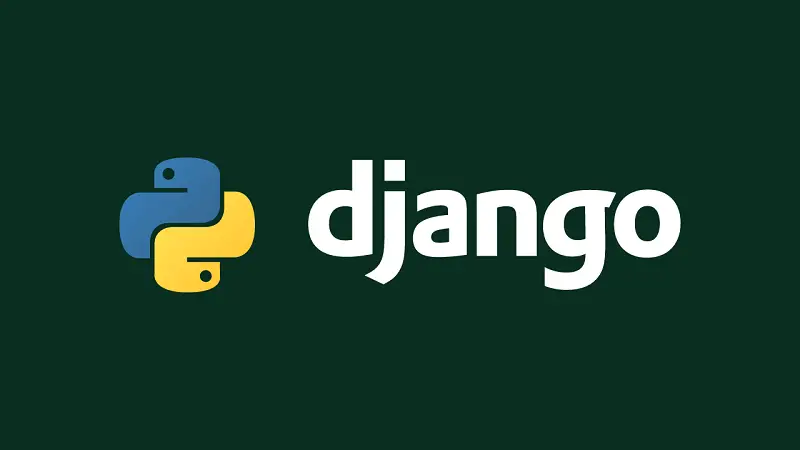
- Summary of what it does: Build web applications
- Creator: Django Software Foundation
- GitHub Stars: 62,117
- Documentation: https://docs.djangoproject.com/
- Official Website: https://www.djangoproject.com/
- GitHub Link: https://github.com/django/django
Django is more of a framework than it is a module because of the great features and tools that come with it.
Django is a high-level Python web framework, it’s very heavyweight and it’s actually used by some major companies like Instagram and Tinder.
Django can be used with other languages and frontend frameworks like React JS, Angular JS, and Vue JS.
It comes with a bunch of tools and complex features that will allow you to make simple and complex enterprise-level websites.
A Django website consists of a single project that is divided into separate apps. Each individual app is considered a Django app and it handles a particular functionality of the website.
Flask
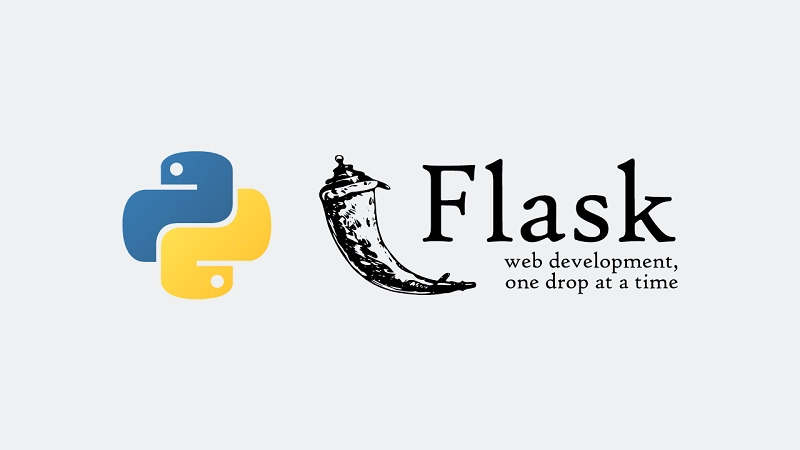
Summary of what it does: Build web applications
Creator: Armin Ronacher
GitHub Stars: 57,819
Documentation: https://flask.palletsprojects.com/
Official Website: https://palletsprojects.com/
GitHub Link: https://github.com/pallets/flask/
The next module I want to talk about is Flask, which is kind of a competitor of Django and FAST API although they have some fundamental differences.
Django, FAST API and Flask work similarly for basic websites although Flask is much easier and faster to get up and running with.
It’s a much lighter-weight web framework and doesn’t come with all of the tools and complex features that are included in Django.
If you are trying to make a very serious or complex website that includes complex user authentication and authorization then you should probably use Django but if you’re doing more of a side project or a simple website then you should pick Flask because Flask is much easier to work with.
Twisted
- Summary of what it does: Build online games
- Creator: Twisted Matrix Laboratories
- GitHub Stars: 4,474
- Documentation: https://twistedmatrix.com/
- Official Website: https://palletsprojects.com/
- GitHub Link: https://github.com/twisted/twisted
Twisted is an event-driven framework for SMTP, HTTP, Proxy, and SSH servers. It allows developers to create servers in minutes without having to use traditional threading models. It is also known for building online games.
Twisted has a huge library of event-based utility classes for managing programming tasks and common protocols, which also includes user authentication and remote object brokering.
Essentially it allows communication between clients and servers and it makes your life a lot easier since you don’t have to reinvent the wheel by building your own socket server from scratch.
If you are trying to build an online interaction or online game then you can include Twisted in your stack.
Beautifulsoup4
- Summary of what it does: Scrape Web pages
- Creator: Leonard Richardson
- GitHub Stars: 4,474
- Documentation: https://www.crummy.com/software/BeautifulSoup/bs4/doc/
- GitHub Link: https://github.com/wention/BeautifulSoup4
The next module on our list is Beautifulsoup4, I believe there are some other versions of this as well.
Beautifulsoup is a great module for scraping the web, if you are doing web scraping; you are trying to scrape some HTML data from a web page then Beautifulsoup4 can do that for you and it’s pretty easy to get that working.
from bs4 import BeautifulSoup
soup = BeautifulSoup("- Somebad HTML
")
print(soup.prettify())
Selenium
- Summary of what it does: Performing Browser Automations
- GitHub Stars: 22,703
- Documentation: https://www.selenium.dev/documentation/
- Official Website: https://www.selenium.dev/
- GitHub Link: https://github.com/SeleniumHQ/selenium
The last module on my list for web development is Selenium.
Selenium is used to perform automation on websites, works on all major OS and its scripts can be written in any programming language like Python, Java, C#, and so on.
It allows you to build browser bots that can interact with other websites.
With Selenium, you can write a script to move your mouse cursor to any position on the browser, perform click events, get buttons and also select HTML fields.
Data Science
The next category to dive into is data science. Python is very popular for Data Science because there are enormous different modules available to make a data scientist’s life much easier.
Numpy Python Module
- Summary of what it does: Performing Mathematical Calculations
- GitHub Stars: 19,519
- Documentation: https://numpy.org/doc
- Official Website: https://numpy.org/
- GitHub Link: https://github.com/numpy/numpy
Numpy is a powerful Python module that can perform any kind of mathematical operation in Python.
Essentially, Numpy is a library that helps in mathematical and numerical computations and operations; and data science programming.
Its powers begin to shine when it’s working with array-like objects in multi-dimensional and matrix multiplications. It is very fast because a lot of its implementations are written in C language.
import numpy as np
a = np.zeros((3,4,5))
print(a)
In brief, Numpy is a tool consisting of numerous functions like array functions that can help you perform any kind of mathematical calculations in Python.
Pandas Python Module
- Summary of what it does: Reading and Working with data frames
- GitHub Stars: 32,548
- Documentation: https://pandas.pydata.org/pandas-docs/stable/
- Official Website: https://pandas.pydata.org/
- GitHub Link: https://github.com/pandas-dev/pandas
Pandas is a well-known open-source library that allows you to perform data analysis and manipulations in Python. Pandas provide an intuitive way to create and manipulate data and it’s built on top of the NumPy package.
With Pandas, you can explore a CSV file, extract the dataset from that CSV into DataFrame (a table) and perform various statistical operations like finding the min, max, average, median, and also clean the data by doing things like filtering rows and columns.
Matplotlib Python Module
- Summary of what it does: Data visualization
- GitHub Stars: 14,975
- Documentation: https://matplotlib.org/stable/api/index
- Official Website: https://matplotlib.org/
- GitHub Link: https://github.com/matplotlib/matplotlib
Matplotlib is a popular Python package for data visualization and it’s built on NumPy arrays, and designed to work with SciPy stack.
Matplotlib library has an object-oriented interface that allows us to create a top-level object to which one or more axes objects are attached.
New data visualization tools like ggplot and ggvis, including web visualization toolkits that are based on HTML5 canvas and D3js, often make Matplotlib feel bulky and old-fashioned.
In brief, Matplotlib is good for visualizing data, making plots and charts, and also works well with machine learning models.
NLTK Python Module
- Summary of what it does: Natural Language Processing
- GitHub Stars: 10,406
- Documentation: https://www.nltk.org/
- GitHub Link: https://github.com/nltk/nltk
NLTK is a toolkit built for working with Natural Language Processing in Python. It is mostly used for text processing and creating visualizations based on the analysis.
Some of the text processing includes tokenization, stemming, lemmatization, lowercase conversion, syntax tree generation, and POS tagging.
OpenCV
- Summary of what it does: Computer Vision
- GitHub Stars: 2,512
- Documentation: https://www.nltk.org/
- GitHub Link: https://github.com/opencv/opencv-python
OpenCV is an open-source library for computer vision, machine learning, image processing, and video processing.
It can do things like license plate reading, robotic vision, facial detection and recognition, image and video processing, optical character recognition, and so on.
It can be integrated with other libraries like Numpy and Matplotlib to make Python capable of processing the OpenCV array structure for analysis.
Machine Learning and AI
Machine learning and Artificial Intelligence are very closely related and connected. Machine learning is a subfield of Artificial Intelligence (AI) and can be considered the application of AI.
TensorFlow Python Module
- Summary of what it does: Deep Learning
- GitHub Stars: 162,623
- Documentation: https://www.tensorflow.org/guide
- Official Website: https://www.tensorflow.org/
- GitHub Link: https://github.com/tensorflow/tensorflow/
TensorFlow is an open-source and popular deep learning library developed by Google.
Deep learning is a subfield of machine learning, a set of algorithms, and is inspired by the structure and function of the human brain.
The TensorFlow library can be used for numerical computations, creating neural networks, and running standard machine learning algorithms.
To understand and work with TensorFlow you need to have a good working knowledge of algebra and vectors.
Keras Python Module
- Summary of what it does: Access TensorFlow Features in an easier way
- GitHub Stars: 53,874
- Official Website: https://keras.io/
- Documentation: https://keras.io/api/
- GitHub Link: https://github.com/keras-team/keras/
Keras is a deep learning API written in Python and can be considered as a layer of abstraction for TensorFlow.
It allows beginners to easily get up and running with deep learning if their goal is to use TensorFlow since it provides TensorFlow features in an easier way.
Pytorch Python Module
- Summary of what it does: Deep Learning
- GitHub Stars: 53,793
- Official Website: https://pytorch.org/
- Documentation: https://pytorch.org/docs/stable/index.html
- GitHub Link: https://github.com/pytorch/pytorch
PyTorch is an optimized tensor library for deep learning using GPUs and CPUs. PyTorch is an open-source deep-learning library developed and maintained by Facebook.
It is primarily used for building Natural Language Processing systems.
Graphical User Interfaces in Python
PyQt5 Module
- Summary of what it does: Building Graphical User Interface.
- GitHub Link: https://github.com/PyQt5
PyQt5 is a package that allows one to use the Qt GUI framework from Python. With the PyQt5 package, you can build a graphical user interface in Python and also use CSS to style your application.
str1 = (“”” bro thanks for the article and your website it’s really seem’s brilliant literally from canada”””)
print(str1, “a good website “)
Developers whose main language is python must know these modules as well.
If he/she doesn’t know theses modules, they are not Python developers.
Hi Maksym, I appreciate your support, but please stop leaving comments on all the articles you visit.