Python is a powerful language. It’s relatively easy to learn and has a steep learning curve. Its design philosophy incorporates modules, dynamic typing, exceptions, classes, and high-level dynamic types.
Python String Methods can save you a lot of hustle when manipulating strings in your project to suit your need. With this in mind, Python comes with around 47 built-in string methods.
The enormous selection of libraries also contributes to the popularity of Python.
Python string is a sequence of Unicode points that is surrounded by quotation marks. Strings are built-in type sequences that can be used to handle textual data in Python.
Note: Python Strings are immutable sequences of Unicode characters.
String literals are written in a variety of ways:
- Single quotes:
'allows embedded "double" quotes'
- Double quotes:
"allows embedded 'single' quotes"
- Triple quoted:
''' Three single quotes '''
,""" Three double quotes """
In this article, we will go over all the built-in Python String Methods. Some of them might be familiar to you but trust me you will also see some rare ones.
Note: All the string methods do not change the original string instead return a copy with the changed attributes.
String Methods, Substrings, And String Interpolation
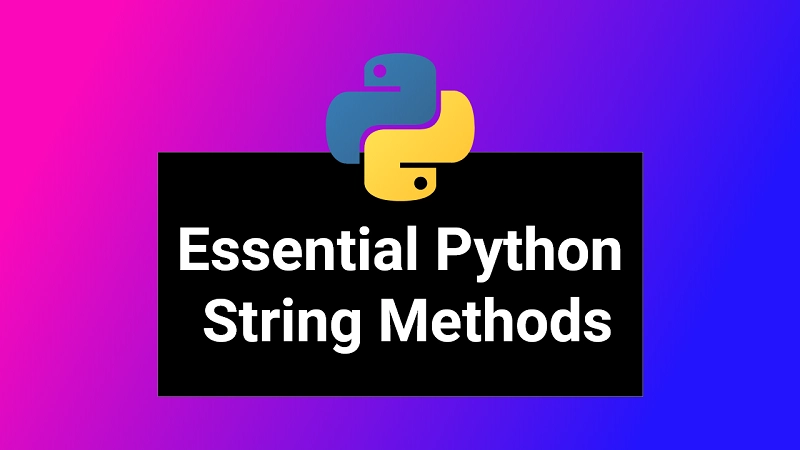
Built-in functions that operate on strings
Python has a substantial number of built-in functions that operate on strings. Note: In Python, built-in functions are predefined functions built into the interpreter and are always available for use anywhere in your Python project or terminal without importing them.
These predefined functions in Python are not specific to string only but may be applicable to other sequence types(list, tuple, set, dict, range) and even other data types.
Don’t get overwhelmed, we are only going to talk about how the built-in functions can be used to manipulate strings.
The table below demonstrates how some of the built-in functions in Python are used on strings.
Python built-in Functions that manipulate string objects
Function | Function Description |
---|---|
len() | Returns the number of elements in the string. Whitespaces are also considered elements in the string. |
slice([start,]stop[,step]) | returns a part of the string |
type() | returns the class type of the object passed as the argument. |
print(s,[, end=None]) | Prints the string into the console. |
int(s[, base=10]) | Takes two parameters: the string to convert into an integer and the optional base you want your result to be represented in. Returns the integer value of the string. |
float([s]) | Returns the floating-point value of the string |
min(s[,default=obj, key=func]) | Returns the minimum value in the string. |
max(s[,default=obj,key=func]) | Returns the maximum value in the string. |
hash(s) | Returns a hash value for the string. |
map(func, s) | Returns a generator object after applying the given function to each item of the iterable. |
id(s) | Returns a unique identity of an object. |
enumerate(s[, start=0]) | Returns an enumerated object that contains each element of the string and its index position in the string into a tuple. |
Cheat Sheet of Python String Methods
This table is a cheat sheet of the Essential Python string methods
Method Name | Method Description |
---|---|
capitalize() | Converts the first character of a string to a capital letter |
casefold() | Converts a string to a lowercase string. This method is an aggressive lower() method that converts a string to a case folded string and also implements caseless string matching. |
center() | Returns a string padded with the specified character. |
count() | Returns the number of occurrences of a substring in the given string |
encode() | Encodes a string with the specified encoding scheme and returns an encoded version of a string. |
endswith() | Returns “True” if the string ends with the given character or substring |
expandtabs() | Specifies a tab size that needs to be substituted with the “\t” symbol in the string and returns it |
find() | Returns the index of the first occurrence of the specific character or substring. |
format() | Formats a string in a nicer way by embedding values into it and returns the result. |
format_map() | Formats specified values in a string using a dictionary |
index() | Returns the index of the first occurrence of the specific character or substring. |
isalnum() | Checks if all the characters of the string are alphanumeric |
isalpha() | Checks if all the characters in the string are alphabets |
isdecimal() | Returns “True” if all the characters in a string are decimal numbers |
isdigit() | Returns “True” if all the characters in the string are numeric digits. |
isidentifier() | Checks if the string is an identifier |
islower() | Checks if all the characters in a string are lowercase |
isnumeric() | Checks if all the characters in a string are numeric |
isprintable() | Checks if all the characters in a string are printable |
isspace() | Returns “True” if all characters in the string are whitespaces |
istitle() | Returns “True” if the first character of the string is a capital letter |
isupper() | Returns “True” if all characters of a string are uppercase |
join() | Returns a concatenated String |
ljust() | Left aligns the string according to the width specified |
lower() | Convert a string to lowercase |
lstrip() | Returns the string with leading characters removed. |
maketrans() | Returns a translation table of a string |
partition() | Breaks the string into parts at the first occurrence of the separator |
replace() | Replaces all occurrences of a substring with another substring and returns the result |
rfind() | Returns the last index at which a specified character or substring was found. |
rindex() | Returns the last index at which a specified character or substring was found. |
rjust() | Right aligns the string according to the width specified. |
rpartition() | Split the given string into three parts. |
rsplit() | Split the string from the right by the specified separator and returns the parts as a list. |
rstrip() | Returns a string with the trailing characters removed. |
splitlines() | Splits a string at line breaks and returns the lines as a list |
startswith() | Returns “True” if a string starts with the specified character or a substring |
strip() | Returns the string with both leading and trailing characters removed |
swapcase() | Converts all uppercase characters to lowercase and vice versa. Case swapping. |
title() | Converts each word of a string to start with a (capital) or uppercase letter |
translate() | Returns a translated string |
upper() | Converts all lowercase characters in a string to upper case |
zfill() | Prefills a string with 0 values |
Thanks for your all essential Python string methods tutorial.
It would be great if you add one more extra.
Using fstring in python is very effective method, you know.
I think this might be added in this list.
print(f”{user.firstname} {user.lastname}”)
Thanks for your effort.
Thanks for the suggestion!