If you are a newbie to JavaScript you may have heard of the DOM (Document Object Model). But what exactly is the DOM?
As a developer, whether you are working on projects with frameworks or building a script from scratch with pure JavaScript, there are some essential DOM Manipulation methods you should be comfortable with.
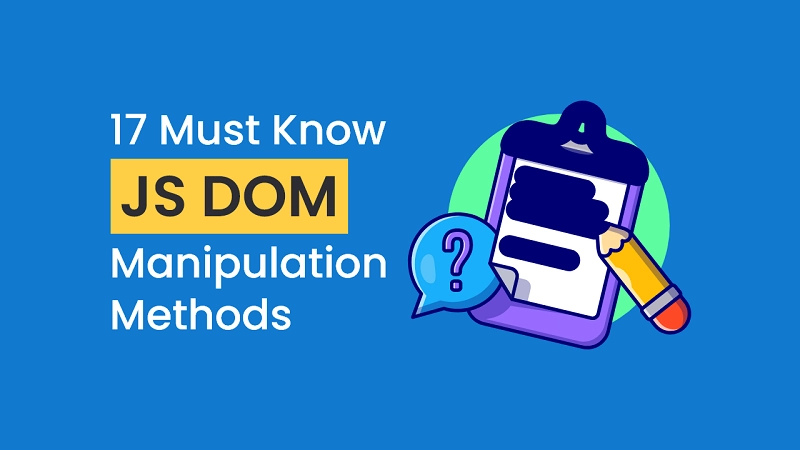
In Understanding How to Manipulate the DOM, we went over how to use built-in methods and properties of Nodes and Elements to manipulate the DOM.
However, frameworks like React Js and Vue Js use virtual DOM to manipulate the Real DOM, whereas Angular Js or Angular also uses incremental DOM to manipulate the Real DOM.
Manipulating the Real DOM is a lot slower than most JavaScript operations. This slowness is made worse since most JavaScript frameworks manipulate the DOM much more than they have to.
To address this problem Angular Js, Vue Js, and React Js popularize something called Virtual DOM and Incremental DOM.
Manipulating the Virtual DOM or Incremental DOM is much faster since nothing gets drawn on the screen.
In your job or if you are a freelancer, you may have to work on existing scripts rather than new projects. So you may not be allowed to use frameworks, libraries or tools.
Knowing these DOM manipulation methods in JavaScript can be really helpful in your career.
In this article, you are going to learn the essential JavaScript DOM manipulation methods with examples.
What is the DOM?
DOM stands for Document Object Model. It is an application programming interface that allows us to create, add, modify and remove elements from the document.
Every page you see in a browser window can be considered as an object. A Document object represents the HTML document that is displayed in that browser window.
The way a document’s content is accessed and modified is called the DOM (Document Object Model).
In brief, the DOM defines the logical structure of a document and the way a document can be accessed and manipulated.
Knowing these DOM manipulation methods can take your JavaScript skills to the next level.
Creating Elements in the DOM
createElement()
The createElement() method creates a new HTML element within the DOM. The return value is the newly created element.
Syntax: document.createElement(tagName[, options])
tagName: A string that specifies the type of HTML element to be created. It can be any of the HTML tags you know about.
options: An optional parameter that enables us to set an “is” attribute when adding an element.
Basic Example
What would it look like to create a paragraph?
/* Create a new paragraph element */
let p = document.createElement('p');
Create a new div element
/* Create a new div element */
let div = document.createElement('div');
What we are actually doing is creating a new element and assigning the returned created element to a variable.
Adding Elements to the DOM
createTextNode()
The createTextNode() method creates a new text node. This method can be used to escape HTML characters.
Syntax: let text = document.createTextNode(data);
text: is a text node
data: a string containing the content to be put in the text node.
Basic Example
// Create a new Text Node
let question = document.createTextNode('How many frameworks do you know?');
Node.textContent
The textContent property of a Node can be used to retrieve or write text inside a Node.
textContent gets the content of all elements including <script> and <style> elements.
Basic Example
Now let’s create a div and add a text of Hello World.
/* Create a new div Element */
let div = document.createElement('div');
/* Add the text Hello World */
div.textContent = 'Hello World';
textContent returns every element in a Node. It can return “hidden” elements (elements having display none) since it does not take CSS Styles into account.
HTMLElement.innerText
HTMLElement.innerText is a property that represents the rendered text content of a node and its descendants.
Basic Example
Let’s create a div and add the text Hello World
/* Create a new div Element */
let div = document.createElement('div');
/* Add the text Hello World */
div.innerText = 'Hello World';
HTMLElement.innerText returns the text content that is displayed on the screen.
append()
The append() method inserts a set of elements or texts after the last child of the Element.
Syntax: append(...nodesorDOMStrings)
Basic Examples
Create a div and append it to the body
/* Get the body */
const body = document.body;
/* Create a new div element */
const div = document.createElement('div');
/* Append the div */
body.append(div);
Create a div then add some text to it and append it to the body
/* Get the body */
const body = document.body;
/* Create a new div element */
const div = document.createElement('div');
/* Add a new text node */
div.textContent = 'Am a developer';
// Append the div
body.append(div);
Create a div and paragraph with texts and append them to the body
/* Get the body */
const body = document.body;
/* Create a new div and paragraph elements */
const div = document.createElement('div');
const p = document.createElement('p');
/* Add text to elements */
div.textContent = 'Am a developer';
p.textContent = 'Hello World';
/* Append the div and paragraph to the body */
body.append(div, p);
appendChild()
The appendChild() method adds a Node after the last child of a specified parent node.
Syntax: appendChild(aChild);
aChild the child to append to the given parent node
Basic Examples
Create a div, add a Hello World text and append it to the body
/* Get the body */
const body = document.body;
/* Create a new div */
const div = document.createElement('div');
/* Add the text Hello world */
div.textContent = 'Hello world';
// Append the div to the body
body.appendChild(div);
Create a heading 1 (h1), add the text Hello World and append it to the body
/* Get the body */
const body = document.body;
/* Create heading 1 (h1) */
const h1 = document.createElement('h1');
/* Add the text Hello world */
h1.textContent = 'Hello world';
/* Append the h1 to the body */
body.appendChild(h1);
appendChild() only accepts one argument of a Node but not DOMstring or text.
innerHTML
The Element property innerHTML retrieves or writes HTML or XML markup contained within the element.
Syntax: const content = element.innerHTML; element.innerHTML = content;
Basic Examples
Suppose that you have the following HTML markup:
<ul id="navList">
<li><a href="">Home</a></li>
<li><a href="">About</a></li>
<li><a href="">Contact</a></li>
</ul>
Let’s use the innerHTML property to get the content of the <ul>
element:
/* Get the ul by it's id */
const menu = document.getElementById('navList');
/* Get the ul content with innerHTML */
console.log(menu.innerHTML);
How it works:
- First select the <ul> element by it’s id (navList) from the DOM with
getElementById()
method. - Now get the HTML content of the <ul> element with innerHTML property
Output:
<li><a href="">Home</a></li>
<li><a href="">About</a></li>
<li><a href="">Contact</a></li>
Suppose we now need our menu to also have a Footer link, then we can append the HTML element for the Footer link to the <ul> element.
/* Get the ul by it's id */
const menu = document.getElementById('navList');
/* Append the HTML for the Footer Link to the ul element */
menu.innerHTML += `<li><a href="">Contact</a></li>`;
Note: Using innerHTML to insert text into a web page can become an attack vector on the site.
HTML5 specifies that a <script> element inserted with innerHTML should not execute.
Node.insertBefore()
The insertBefore() method inserts a node as a child of some specified parent node before the referenced node.
Syntax insertBefore(newNode, referenceNode);
Basic Examples
Suppose that you have the following HTML markup:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<script src="./test.js" defer></script>
<title>createElement</title>
</head>
<body>
<div id="parentElement">
<div id="childElement">Am a div</div>
</div>
</body>
</html>
Then, let’s use insertBefore() to insert a heading 1 (h1) before the first child of the parent element.
/* Get parent div by it's id */
const parentElement = document.getElementById('parentElement');
/* Create heading 1 (h1) element */
const h1 = document.createElement('h1');
/* Add a text of "My First JavaScript Project" to the h1 element */
h1.textContent = 'My First JavaScript Project';
/* Insert the h1 before the first child of the parentElement */
parentElement.insertBefore(h1, parentElement.firstChild);
How it works
- Get the parent div with getElementById() method from the DOM
- Then create an h1 element with createElement() method
- Now, insert text into the created h1
- Finally, insert the <h1> before the first child of the parentElement
insertAdjacentHTML()
The insertAdjacentHTML() method of the Element interface parses the specified text as HTML or XML markup and inserts the resulting nodes into the DOM tree at a specified position.
Syntax element.insertAdjacentHTML(position, text);
position – A string representing the position relative to the element.
'beforebegin'
: Before theelement
itself.'afterbegin'
: Just inside theelement
, before its first child.'beforeend'
: Just inside theelement
, after its last child.'afterend'
: After theelement
itself.
Better visualization
<!-- beforebegin --> <p> <!-- afterbegin --> foo <!-- beforeend --> </p> <!-- afterend -->
It is not recommended to use insertAdjacentHTML() method when inserting plain text, use the Node.textContent
property or the Element.insertAdjacentText()
method.
Modifying Data Attibutes in the DOM
getAttribute()
The getAttribute() method of the Element interface returns the value of a specified attribute on the element.
Syntax const attribute = element.getAttribute(attributeName);
Suppose you have this HTML
<!DOCTYPE html>
<html lang="en">
<head>
<title>Data Attribute</title>
</head>
<body>
<div id="data">My first Javascript Project</div>
</body>
</html>
Then let’s use getAttribute() to get the value of the attribute id of the <div>.
// in a console
const div1 = document.getElementById('data');
//=> My first Javascript Project
const exampleAttr = div1.getAttribute('id');
//=> "data"
const title = div1.getAttribute('title');
//=> null
setAttribute()
The setAttribute() method sets an attribute on a specified element.
Syntax Element.setAttribute(name, value);
Basic Example
Let’s use the HTML we used in the getAttribute() example
Now, we need to set an attribute called name and give it a value of john
/* Get the div element */
const div1 = document.getElementById('data');
/* Set the attribute name with a value of john on the div */
div1.setAttribute('name', 'john')
How it works
- First we get the element we want to set the attribute on from the DOM tree with getElementById() method
- Next, we call the setAttribute() method on the element and pass in two arguments, the first argument is the name of the attribute and the second is the value of the attribute.
Element.dataset
The Element.dataset property provides read and write access to custom data attributes (data-*) on elements.
Syntax const dataAttrMap = element.dataset
Basic Example
Suppose you have this HTML
<div id="user">By his Grace</div>
Let’s get the <div> by its id and add two datasets (dataset-id and dataset-name) to it.
/* Get the div by its id */
const user = document.getElementById('user');
/* Add two datasets (dataset-id and dataset-name) */
user.dataset.id = '1234';
user.dataset.name = 'John Doe';
How it works
- First we select the element we want to add the datasets to
- We use
Element.dataset.property
to assign the datasets to the element
Output HTML
<div id="user" data-id="1234" data-name="John Doe">By his Grace</div>
Element.classList
classList is a property of an element that holds the class names of the element currently selected. The classList property also returns an array of the classes on the selected element.
Suppose you have this HTML in the body tag
<div id="navigation" class="navbar active">
<ul>
<a href="">Home</a>
<a href="">About</a>
<a href="">Search</a>
</ul>
</div>
You can select the navigation div by its id with the getElementById() method, then you can fetch the class names of the element using either className or classList property.
The className property returns the class names as a string whilst the classList property returns the class names as an array.
// Get the navigation by id
const navigation = document.querySelector('#navigation');
console.log(navigation.className); //=> "navbar active"
console.log(navigation.classList); //=> ["navbar" "active"]
console.log(navigation.classList[0]); //=> "navbar"
console.log(navigation.classList[1]); //=> "active"
The classList property is read-only so if you want to manipulate the HTML element’s classes, you will need to use the methods that come with the classList property.
- add()
- contains()
- remove()
- replace()
- toggle()
classList add() method
The classList.add() method allows you to add at least one class name to an element, omitting any that are already present.
Here’s an example of adding a new class name to the navigation <div> element above:
/* Get the navigation by id */
const navigation = document.querySelector('#navigation');
/* Add a single class to the navigation div */
navigation.classList.add('open');
Here’s an example of adding multiple class names to the navigation <div> element above:
/* Get the navigation by id */
const navigation = document.querySelector('#navigation');
/* Add a single class to the navigation div */
navigation.classList.add('open', 'close', 'greet', 'salute');
classList contains() method
The classList.contains() method check on the element’s class attribute to see if it contains the specified class name.
It accepts the class name to be checked as an argument. The method will return true if the specified class is present or false if it’s not present.
/* Get the navigation by id */
const navigation = document.querySelector('#navigation');
/* Add a single class to the navigation div */
navigation.classList.contains('navbar'); //=> true
navigation.classList.contains('myBoy'); //=> false
In brief, the contains() method return a boolean.
classList remove() method
The classList.remove() method allows you to remove one or more class names from an HTML element.
Similar to the add() method, the remove() method also accepts at least one string argument separated by commas.
/* Get the navigation by id */
const navigation = document.querySelector('#navigation');
/* remove three class names */
navigation.classList.remove('navbar', 'open', 'close');
When removing a class that doesn’t exist JavaScript won’t throw an error.
classList toggle() method
The classList.toggle() method allows you to add or remove a class name based on the current class value on the element.
If the class name already exists on the element, the toggle() method will remove that class name and vice versa.
Suppose you have an <h1> element with some text
<h1 id="color" class="pink">Please choose a color</h1>
You can call the toggle() method on the heading to remove the class “pink” from the element.
/* Get the heading element for the DOM */
const heading = document.getElementById('color');
heading.classList.toggle('pink'); //=> false
heading.classList.toggle('pink'); //=> true
When the toggle() method removes a class, it will return false. Also, when it adds a class, it will return true.
Removing Elements from the DOM
remove()
The Element.remove() method removes the element from the DOM.
Syntax remove()
Suppose we have this HTML
<!DOCTYPE html>
<html lang="en">
<head>
<title>Data Attribute</title>
</head>
<script src="./test.js" defer></script>
<body>
<div id="blue">Blue books</div>
<div id="orange">Orange mangoes</div>
</body>
</html>
Then we want to remove the element with the id attribute of orange
/* Get the div element */
const orange = document.getElementById('orange');
/* Remove the element from the DOM */
orange.remove();
How it works
- First we get the element to be removed from the DOM with getElementById() method
- Then, we call the remove() method on the element to remove it.
removeChild()
The removeChild() method removes a child node from the DOM and returns the removed node.
Syntax removeChild(child);
Suppose we have this HTML
<div class="colors">
<div id="blue">Blue books</div>
<div id="orange">Orange mangoes</div>
</div>
Then we want to remove the orange div from the colors div using removeChild()
/* Get the div element */
const orange = document.getElementById('orange');
/* Remove the child node from the DOM */
if (orange.parentNode) {
orange.parentNode.removeChild(orange);
}
How it works
- First we get the orange element from the DOM
- Then we use an if statement to check if it has a parent
- Finally, if that condition is true then we call the removeChild() method on the orange parent div.
Totally agree that mentioned DOM manipulation methods are the most important and must things.
Thanks for your post.